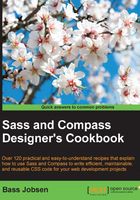
Interpolation of variables
Variable interpolation means the ability to build a string by using the values assigned to variables. In Sass, you can use variable interpolation to dynamically create property and selectors' names. In this recipe, you will learn how to apply variable interpolation in your code.
Getting ready
You can test variable interpolation in Sass with the Ruby Sass compiler. The Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, describes how to install Ruby Sass on your system.
How to do it...
Perform the steps beneath to learn how to use variable interpolation in Sass to dynamically create property and selector names:
- Write down the Sass code as follows in a Sass template called
interpolation.scss
:$name: class; $direction: left; $units: px; .#{$name} { margin-#{$direction}: 20#{$units}; }
- Compile the Sass template from the previous step with Ruby Sass by running the following command in your console:
sass interpolation.scss
- The compiled CSS code from the first three steps should look like that shown here:
.class { margin-left: 20px;
How it works...
In Sass, you can use string interpolation by using the #{}
interpolation syntax. The syntax enables you to use SassScript variables in selectors' and property names and values.
SassScript supports quoted and unquoted strings. In SassScript, quoted strings are compiled into quoted strings. But when you're using quoted strings for interpolation, the value is unquoted first. Consider the following SCSS code:
// scss-lint:disable PropertySortOrder, PropertySpelling $value1: unquoted; $value2: 'quoted'; test { p1: $value1; p2: $value2; p3: $value1 #{$value2}; p4: $value1#{$value2}; p5: $value1 + #{$value2}; p6: $value1 + $value2; p7: $value1 $value2; }
The preceding Sass code compiles into CSS code as follows:
test { p1: unquoted; p2: "quoted"; p3: unquoted quoted; p4: unquotedquoted; p5: unquoted + quoted; p6: unquotedquoted; p7: unquoted "quoted"; }
In the preceding CSS code, you can see that the quotes are removed from the $value2
variable for the p3
, p4
, and p5
properties when applying string interpolation with the #{}
interpolation syntax. For the p5
property, the +
operator has not been applied, while the p6
and p7
properties will show how the strings are concatenated, as described in the Applying operations in Sass recipe of this chapter.
There's more...
Other use cases of variable interpolation can be found elsewhere in this book too. In the Commenting your code in the SCSS syntax recipe of Chapter 2, Debugging Your Code, you can read how to use variable interpolation to generate copyright notices in your CSS code. The Applying operations in Sass recipe of this chapter describes why you should use variable interpolation when using the calc()
CSS function in your code.
Variable interpolation can also be used inside mixins and functions to dynamically compile a list of selectors or values. You can read more about mixins in the Leveraging mixins and Writing mixins with arguments recipes of this chapter.