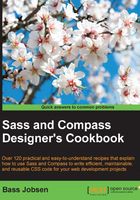
Declaring variables with !default
When you start using frameworks, such as Bootstrap and Foundation, as discussed further on in this book, you will also start using variables for customization. The usage of the !default
keyword when declaring the framework's variables makes it possible to customize the code without changing the original code. Keeping the original code untouched enables you to update the framework source code without destroying your modifications.
Getting ready
Install Ruby Sass as described in the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass. You can edit the SCSS code of this recipe with a text editor.
How to do it...
The following steps will show you how to use the !default operator when declaring variables:
- Create a Sass template called
default.scss
and write down the following SCSS code into this file:// scss-lint:disable ColorKeyword $color: red; $color: green !default; .green { color: $color; }
- Now run the following command in your console:
sass default.scss
- You will find that the command from the previous step outputs the CSS code like that shown here into the command line:
.green { color: red; }
- When you remove the first
$color: red;
declaration in thedefault.scss
file, the defaultgreen
value of the$color
variable is used by the compiler. And the compiled CSS code will look as follows:.green { color: green; }
How it works...
If you are writing reusable code for your project, it will make sense to define your variable in a single place. When you use a variable before declaring it, the compiler will throw an Undefined variable
error and stop the compilation process. So, your code should have default values for the variables used for customization. In Sass, you can create default variable values with ease by putting the !default
keyword after the variable's value. Your variable's declaration with a default value should look as follows:
$variable: value !default;
To override a default value, you should declare a variable with the same name and a different value. Because the default values are used elsewhere in your code, you should override them before or directly after their declaration. If you override your default value after using it, any usage before the override will get the original default value. In case your default variables are declared in a partial file, you should declare your overriding variable before the declaration of the @import
directive. You can learn more about the @import
directive in the Importing and organizing your files recipe of Chapter 9, Building Layouts with Sass.
The example code in this recipe defines all the Sass code in a single file. In practice, your default variables will be declared in one or more partials. You can read more about partials in the Working with partials recipe of Chapter 1, Getting Started with Sass.
Consider the situation that you got a partial called _default-variables.scss
that contains, for instance, the following code:
$color: red !default;
Now, you can create a main.scss
file for your project, which will contain the following code:
// customization $color: green; // default variables @import "default-variables" // import other code that uses the variables defined above here
The compiler will use green now for every $color
usage in your code. You can easily change the $color
variable without having to change the _default-variables.scss
file. If the _default-variables.scss
file contains many variables, you will have to override only the variables you want to change.
There's more...
In the There's more… section of the Using variables recipe of this chapter, you can read about the variable's scope in Sass. You can also use the !default
keyword in a local scope so that the global scope can override the default value. In the following example, you can see that the local scope inside the .link
selector used the color value assigned to the $color
variable in the global scope:
$color: red; .link { $color: green !default; color: $color; }
The preceding SCSS code compiles into CSS code as follows:
.link { color: red; }
In Chapter 12, Bootstrap and Sass, of this book, you can read about Bootstrap. All Bootstrap's variables are defined with the !default
keyword in a single partial file called _variables.scss
. Foundation, as described in Chapter 11, Foundation and Sass, also uses a special _settings.scss
partial file with default variables.