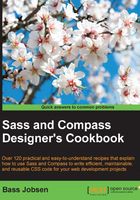
Leveraging mixins
Mixins play an important role in Sass and help you write reusable code and use semantic HTML code.
Mixins are like macros and allow you to reuse properties and selectors. People used to functions programming will tend to see mixins like functions. Mixins do not return a value at runtime, but generate an output at compile time. For functions in Sass, read the Creating pure Sass functions recipe of this chapter.
Getting ready
You can test the mixins in this recipe with the Ruby Sass compiler. The Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, describes how to install Ruby Sass on your system.
How to do it...
The steps beneath will help you to understand how to use mixins in your Sass code:
- Create a Sass project file, which contains the following code:
@import 'components/responsive-utilities'; // scss-lint:disable ColorKeyword $side-bar-background-color: orange !default; .side-bar { @include hidden-mobile; background-color: $side-bar-background-color; li { list-style: square; } } .banner { @include hidden-mobile; img { float: right; } }
- The project file from the first step imports the
components/_responsive-utilitie
s.scss file. This file should contain the following Sass code:@mixin hidden-mobile { @media (max-width: 767px) { // scss-lint:disable ImportantRule display: none !important; } }
- Now, compile your project by running the following command in your console:
sass sass/main.scss
- Finally, the compiled CSS code should look as follows:
.side-bar { background-color: orange; } @media (max-width: 767px) { .side-bar { display: none !important; } } .side-bar li { list-style: square; } @media (max-width: 767px) { .banner { display: none !important; } } .banner img { float: right; }
How it works...
Mixins enable you to define styles, which can be reused everywhere in your code. You can define a mixin with the @mixin
directive. Mixins do not only contain properties, but also selectors and other valid CSS code. You can use SassScript inside your mixins too, which will enable you to dynamically create selectors' and property names. Mixins can also have arguments; you can read more about this in the Writing mixins with arguments recipe of this chapter.
After defining your mixins, you can import them by using the @import
directive. You can read more about the @import
directive in the Importing and organizing your files recipe of Chapter 9, Building Layouts with Sass.
The hidden-mobile
mixin used in this recipe contains a media query. You can read more about the @media
rules and Sass in the Creating responsive grids recipe of Chapter 9, Building Layouts with Sass. By using the hidden-mobile
mixin, you can directly hide your elements on small screens without the need of having an additional .hidden-mobile
class for hiding. You can also apply the mixins to semantic HTML5 elements, such as the header
, footer
, section
, and aside
elements.
When your mixin generates a selector, you can import it in the global scope without the need to nest the @include
directive in a selector. The following example code shows how a mixin can be imported in the document's root:
@mixin half { footer { width: 50%; } } @include half;
This code will compile into CSS as follows:
footer { width: 50%; }
There's more…
CSS' clear fixes are frequently used in layouts with floated elements. The clear fix clears the float of an element's child element without any additional markup.
Bootstrap, as discussed in Chapter 12, Bootstrap and Sass, used the clear fix mixin shown here. The clear fix itself is adopted from http://nicolasgallagher.com/micro-clearfix-hack/:
@mixin clearfix() { &:before, &:after { content: " "; // 1 display: table; // 2 } &:after { clear: both; }
You can include this mixin everywhere in your project's code to clear the floats. Notice that mixins are not only reusable in your project, but are portable too. You can easily import the clear fix mixins from Bootstrap in your project by using the @import
directive, as described in the Importing and organizing your files recipe of Chapter 9, Building Layouts with Sass.
Every time that you use the clear fix mixin, the same properties will be copied in your compiled CSS code. Repeating the same properties again and again will make the compiled CSS code larger than strictly necessary. While you are writing modular CSS code, refer to Chapter 4, Nested Selectors and Modular CSS; maybe, you don't care about these additional bytes of code. You possibly prefer the grouping of connected codes together in favor of some duplicate code. If you do not care about the order of the compiled CSS code, you can consider using the @extend
directive instead of a mixin. You can read more about the @extend
directive in the Utilizing the @extend directive recipe of Chapter 4, Nested Selectors and Modular CSS.
See also
To learn more about semantic HTML code, you should read the Let's Talk about Semantics article by Mike Robinson. You can find Robinson's article at http://html5doctor.com/lets-talk-about-semantics/.