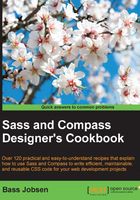
Applying operations in Sass
You can use SassScript to assign a value to a property or variable. SassScript enables you to apply standard arithmetic operations (adding, subtracting, multiplying, dividing, and modulo) when assigning values. Other operations are available per data type. In this recipe, you will learn how to use operations in SassScript to assign values.
Getting ready
For this recipe, you will use the command-line Sass compiler. Read the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, to find out how to install it. Operations with SassScript can easily be tested in the interactive mode of Ruby Sass. In the Using Sass interactive mode and SassScript recipe of Chapter 1, Getting Started with Sass, you can read how to use this interactive mode.
How to do it...
Perform the following steps to find out how to apply operation on assigned values:
- Create a Sass template called
operations.scss
. This file should contain the SCSS code as follows:// scss-lint:disable ColorKeyword, PropertySortOrder, PropertySpelling $color1: blue; $color2: red; $distance: 10px; .operations { color: $color1 + $color2; width: $distance * 100; height: ($distance + 10) * 5; height-2: $distance + 10 * 5; font-family: sans- + 'serif'; }
- Compile the file into the CSS code by running the following command in your console:
sass --watch operations.scss
- Now you will find that the compiled CSS looks as follows:
.operations { color: magenta; width: 1000px; height: 100px; height-2: 60px; font-family: sans-serif; }
How it works...
If you use arithmetic operations to assign values with SassScript, all the common mathematical rules will be applied. Mathematical rules are also applied to the units, as described in detail in the There's more... section of the Creating pure Sass functions recipe of this chapter. Parentheses can be used for precedence rules.
As you can see, operations can not only be applied to numbers, but also to other data types supported by SassScript. SassScript supports seven data types: numbers
, quoted
and unquoted strings
, colors
, booleans
, nulls
, lists
, and maps
. The color: $color1 + $color2;
declaration shows you that you can sum up two colors. For the summation, you can add the color values of each color of the RGB triplet; #ff0000
(red) + #0000ff
(blue) = #ff00ff
(magenta). For colors, ff
is the highest hexadecimal value; color calculations are not circular, so ff + 01 = ff
.
Operations on colors defined with the rgba()
and hsla()
CSS color values can only be applied when the colors have the same alpha channel. Notice that Sass supports its own rgba()
and hsla()
functions too. In Sass, the rgba(blue,0.5)
call compiles into the rgba(0,0,255,0.5)
CSS code. When working with colors, you should possibly prefer the color functions, as described in the Color functions recipe of Chapter 5, Built-in Functions, rather than just applying operations.
The font-family: sans- + 'serif';
declaration shows you how to concatenate string by using the + operator.
You cannot apply operations on lists
and maps
. Also, for these data types, you can use the built-in functions, as described in the List functions recipe and Map functions recipe of Chapter 5, Built-in Functions.
Finally, the height: ($distance + 10) * 5;
declaration also shows you that precedence rules are correctly applied.
Sass math functions preserve units during arithmetic operations. Units must be the same or compatible to preserve them. For example, you cannot mix px
with the em
values. You can read more about unit and arithmetic operations in the There's more… section of the Creating pure Sass functions recipe of this chapter.
There's more...
With the calc()
CSS function, you can perform calculations to determine CSS property values. Nowadays, most of the major web browsers support the calc()
function. The following CSS code will show you a use case for the calc()
function:
div { width: calc(100% - 20px); }
When your Sass code to compile the preceding CSS code contains one or more variables, the compiler tries to apply the operation and solve 100% - 20px
. The preceding operation generates an Incompatible units
error in the first place. Secondly, it should compile into unwanted CSS code. You can solve this issue by using variable or string interpolation. Consider a SCSS code like that shown here:
$border-margin: 20px; div { width: calc(100% - #{$border-margin}); }
The preceding SCSS code will compile into CSS code, as described in the use case at the beginning of this section. You can read more about variable interpolation in the Interpolation of variables recipe of this chapter.
When developing and maintaining style sheets for your website or app, colors are often subjects of change. It seems to be a good practice to use global variables for colors everywhere in your code; this makes it easy to update the color, as you only need to change it at one place. In the Writing your code in a text editor recipe of Chapter 1, Getting Started with Sass, you can read about the scss-lint
tool. This tool checks your Sass code for hard coded color values and throws an error when found.
See also
- The CSS Color Module Level 3, which includes the
rgba()
andhsla()
CSS color values, can be found at http://www.w3.org/TR/css3-color/. - Read more about the
calc()
CSS function at https://developer.mozilla.org/en-US/docs/Web/CSS/calc. - You can counsel the Can I Use database to find out which browsers support a CSS feature or not. You can find this database at http://caniuse.com/.