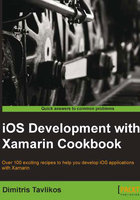
Navigating through different view controllers
In this recipe, we will learn how to use the UINavigationController
class to navigate among multiple view controllers.
Getting ready
The UINavigationController
class is a controller that provides hierarchical navigation functionality with multiple view controllers. Create a new iPhone Empty Project in Xamarin Studio and name it NavigationControllerApp
.
How to do it...
Perform the following steps to create navigation among multiple view controllers:
- Add three new iPhone view controllers in the project and name them
MainController
,ViewController1
, andViewController2
. - Open the
AppDelegate.cs
file and add the following code in theFinishedLaunching
method:MainController mainController = new MainController(); mainController.Title = "Main View"; UINavigationController navController = new UINavigationController(mainController); window.RootViewController = navController;
- Open
MainController.xib
in Interface Builder and add two buttons with their corresponding outlets. Set their titles toFirst View
andSecond View
, respectively. - Add the following code in the
ViewDidLoad
method of theMainController
class:this.buttonFirstView.TouchUpInside += (sender, e) => { ViewController1 v1 = new ViewController1(); v1.Title = "First View"; this.NavigationController.PushViewController(v1, true); } ; this.buttonSecondView.TouchUpInside += (sender, e) => { ViewController2 v2 = new ViewController2();v2.Title = "Second View"; this.NavigationController.PushViewController(v2, true); };
- Add a button in each of the
ViewController1
andViewController2
controllers in Interface Builder with the titlePop to root
. Then, add the following code in both of these controllers'ViewDidLoad
methods:this.buttonPop.TouchUpInside += (sender, e) => { this.NavigationController.PopToRootViewController(true); };
- Run the app on the simulator.
- Click on the buttons and see how the user interface navigates from one controller to another.
How it works...
The UINavigationController
class preserves a stack of controllers. The UIViewController
class has a property named NavigationController
. In normal situations, this property returns null. However, if the controller is pushed into a navigation controller's stack, it returns the instance of the navigation controller. In this case, all of our controllers' NavigationController
property will return the instance of our navigation controller. So this way, at any point in the hierarchy of controllers, access to the navigation controller is provided. To push a view controller to the navigation stack, we call the UINavigationController.PushViewController(UIViewController, bool)
method, using the following line of code:
this.NavigationController.PushViewController (v1, true);
Notice that the MainController
class is the topmost or root controller in the navigation stack. A navigation controller must have at least one view controller that will act as its root controller. We can provide it upon initialization of the navigation controller, as follows:
UINavigationController navController = new UINavigationController(mainController);
To return to the root controller, we call the PopToRootViewController(bool)
method inside the current controller, as follows:
this.NavigationController.PopToRootViewController (true);
The boolean parameters in both methods are used for animating the transition between the view controllers. Setting it to false
will result in the controllers instantly snapping onto the screen, which in most cases does not provide a very good user experience.
There's more...
In this simple example, we provided backward navigation to the root controller with buttons. Notice that there is an arrow-shaped button at the top bar, as shown in the following screenshot:

This top bar is called the navigation bar and is of the UINavigationBar
type. The arrow-shaped button is called the back button and is of the UIBarButtonItem
type. The back button, when it exists, always navigates to the previous controller in the navigation stack. If the previous controller in the stack has its Title
property set, the back button will display that title. If it does not have a title, the back button will be titled Back
.
To change, add, and hide the buttons of the navigation bar, we can use the following methods of our currently displayed view controller's NavigationItem
property:
SetLeftBarButtonItem
: This method adds a custom button on the left-hand side of the navigation bar, replacing the default back button.SetRightBarButtonItem
: This method adds a custom button on the right-hand side of the navigation bar.SetHidesBackButton
: This method sets the visibility of the default back button.
To remove or hide the custom buttons on the left or right-hand side of the navigation bar, call the appropriate methods passing null instead of a UIBarButtonItem
object.
See also
- The Modal view controllers and Using view controllers efficiently recipes
- The Animating views recipe in Chapter 11, Graphics and Animation