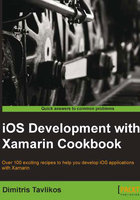
Loading a view with a view controller
In this recipe, we will learn how to use the UIViewController
class to manage views.
Getting ready
Create a new iPhone Empty Project in Xamarin Studio and name it ViewControllerApp
.
How to do it...
Perform the following steps to load a view with a view controller:
- Add a new file to the project.
- Right-click on the project in the Solution pad and go to Add | New File….
- In the dialog that will appear, select iPhone View Controller from the iOS section. Name it
MainViewController
and click on the New button. Xamarin Studio will create a new XIB file and will automatically open theMainViewController.cs
source file. This file contains a class that overrides theUIViewController
class, and we can implement any code related to our view controller in it. - Open the
MainViewController.xib
file in Interface Builder. - Add
UILabel
on the view. - Create and connect an outlet for it inside the
MainViewController
class and name itmyLabel
. - Enter the text
View in controller!
in the label. - Save the XIB document.
- In Xamarin Studio, enter the following code in the
FinishedLaunching
method of theAppDelegate
class, right after the window initialization line:MainViewController mainController = new MainViewController (); window.RootViewController = mainController;
- Compile and run the app on the simulator.
How it works...
When we add a new iPhone View Controller
file in a project, in this case MainViewController
, Xamarin Studio basically creates and adds the following three files:
MainViewController.xib
: This is the XIB file that contains the controller.MainViewController.cs
: This is the C# source file that implements the class of our controller.MainViewController.designer.cs
: This is the autogenerated source file that reflects the changes we make to the controller in Interface Builder.
Notice that we do not need to add an outlet for the view as this is taken care of by Xamarin Studio. We initialize the controller through its class, as follows:
MainViewController mainController = new MainViewController ();
Then, we assign the controller to the window.RootViewController
property, as follows:
window.RootViewController = mainController.
Our view controller is now the root view controller of our app's window, and it is the first one that will be shown when the app starts.
There's more...
The project we have just created only shows how we can add a controller with a view. Notice that we created the outlet for the label inside the MainViewController
class, which acts as the file's owner object in the XIB file. To provide some functionality for the MainViewController
class, add the following method in the MainViewController
class in the MainViewController.cs
file:
public override void ViewDidLoad () { this.myLabel.Text = "View loaded!"; }
This method overrides the UIViewController.ViewDidLoad()
method, which is executed after the controller has loaded its view.
The UIViewController
class contains a number of methods that allow us to manage the view controller's life cycle. These methods are called by the system on the view controller, and we can override them to add our own implementation. Some of these methods are as follows:
ViewWillAppear
: This method is called when the controller's view is about to appear.ViewDidAppear
: This method is called when the controller's view has been displayed.ViewWillDisappear
: This method is called when the controller's view is about to disappear, for example, when another controller will be displayed.ViewDidDisappear
: This method is called when the view has disappeared.
See also
- The Navigating through different view controllers recipe
- The Creating an iOS project with Xamarin Studio and Accessing the UI with Outlets recipes from Chapter 1, Development Tools