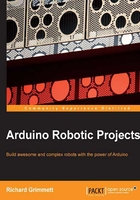
Running the IDE for the Adafruit FLORA
When connecting the FLORA device, you'll need to have the Adafruit version of the IDE installed. You can download this from learn.adafruit.com/getting-started-with-flora/download-software. Follow the directions on this site to download and install the IDE. Since the FLORA device is not standard Arduino, this will add another selection to the Board type for the Arduino IDE.
Installing the Adafruit drivers
When plugging in the device, if it fails to install, you may have to tell it where to find the drivers. You will know if this happens—you will get an error message saying Device driver software was not successfully installed. If you get this error, follow the directions at http://Arduino.cc/en/Guide/Windows#.UxoWXPldUvt; only point your driver to the directory from where you downloaded the Adafruit IDE. For example, in my case, I am running 64 bit Windows, so I will select windows as shown in the following screenshot:

It will probably complain about an unsigned driver, but accept the driver anyway. In the end, you should be able to see the FLORA device when you navigate to Start Menu | Devices and Printers as shown in the following screenshot:

When you select this device and look at its properties, you should see something like the following screenshot:

Note the device COM port; in this case, it is COM25. Now, you should open the Adafruit Arduino IDE by going to the directory where you unzipped the files and select the Arduino IDE. Just a note, since I often use both types of Arduino devices, I have a separate directory for the standard Arduino IDE and a different location for the Adafruit Arduino IDE. To open the Adafruit Arduino IDE, I go to the file browser and select the Arduino IDE executable:

Selecting the Adafruit boards
The Arduino IDE should start and look very much like it did for Arduino Uno or Mega. However, when you select board by going to Tools | Board, you should see four new selections at the bottom:

Selecting the COM port
Now that your board is selected, you'll need to select the COM port. Do this by navigating to Tools | Serial Port | COM25 as shown in the following screenshot:

Coding an LED flash on the FLORA
Now you should be able to upload a file. You can't use the earlier Blink example as we don't have the same I/O pins. So, type the following code into the IDE interface:
// Pin D7 has an LED connected on FLORA. // give it a name: int led = 7; // the setup routine runs once when you press RESET: void setup() { // initialize the digital pin as an output. pinMode(led, OUTPUT); } // the loop routine runs over and over again forever: void loop() { digitalWrite(led, HIGH); // turn the LED on delay(100); // wait for a second digitalWrite(led, LOW); // turn the LED off delay(1000); // wait for a second }
Tip
Downloading the example code
You can download the example code files for all the Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed to you.
Don't worry about specific code details yet, but this code will flash the LED on FLORA. Upload the code by clicking on the Upload button as follows:

When you have uploaded the file, you should get an indication in the lower-left corner of the IDE. Now the red LED should be flashing much faster. You can change the delay(1000)
value and see different flash timing.