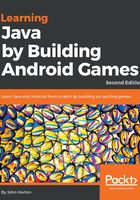
Initializing the objects
Next, just as with regular variables, we need to initialize our objects before using them.
We won't go into great detail about how this next code works until Chapter 8, Object-Oriented Programming, however, just note that in the code that follows each of the objects we made in the previous block of code is initialized.
// Initialize all the objects ready for drawing // We will do this inside the onCreate method int widthInPixels = 800; int heightInPixels = 800; myBlankBitmap = Bitmap.createBitmap(widthInPixels, heightInPixels, Bitmap.Config.ARGB_8888); myCanvas = new Canvas(myBlankBitmap); myImageView = new ImageView(this); myPaint = new Paint(); // Do drawing here
Notice the comment in the previous code.
// Do drawing here
This is where we would configure our color and actually draw stuff. Also, notice at the top of the code we declare and initialize two int
variables called widthInPixels
and heightInPixels
. As I mentioned previously, I won't go into detail about exactly what is happening under the hood when we initialize objects but when we code the Canvas
demo app I will go into greater detail about some of those lines of code.
We are now ready to draw. All we must do is assign the ImageView
to the Activity
.