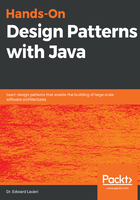
上QQ阅读APP看书,第一时间看更新
Sample OOP class
Let's develop our Bicycle class further by adding attributes and a behavior. The attributes will be the number of gears, how much the bicycle cost, the weight, and the color. The behavior will be the ability to output the attribute values. Here is the source code:
public class Bicycle extends TwoWheeled {
// instance variable declarations
private int gears = 0;
private double cost = 0.0;
private double weight = 0.0;
private String color = "";
// method to output Bicycle's information
public void outputData() {
System.out.println("\nBicycle Details:");
System.out.println("Gears : " + this.gears);
System.out.println("Cost : " + this.cost);
System.out.println("Weight : " + this.weight + " lbs");
System.out.println("Color : " + this.color);
}
Here is the code for Setters:
public void setGears(int nbr) {
this.gears = nbr;
}
public void setCost(double amt) {
this.cost = amt;
}
public void setWeight(double lbs) {
this.weight = lbs;
}
public void setColor(String theColor) {
this.color = theColor;
}
}
We will take a look at all components of the Bicycle class in subsequent sections. For now, focus on the public void outputData() method. We will use our Driver class to evoke that method. Here is the updated Driver class:
public class Driver {
public static void main(String[] args) {
Bicycle myBike = new Bicycle();
myBike.setGears(24);
myBike.setCost(319.99);
myBike.setWeight(13.5);
myBike.setColor("Purple");
myBike.outputData();
}
}
In the preceding Driver class, we create an instance of Bicycle called myBike, assign values to its attributes, and then make a call to the outputData() method. Here are the output results:

Driver class output