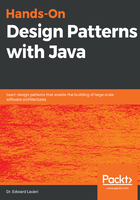
上QQ阅读APP看书,第一时间看更新
Polymorphism
Polymorphism is not a word that non-OOP programmers are likely familiar with. The term polymorphism is generally defined as appearing in multiple forms. In OOP, polymorphism states that different types of objects can be accessed via a common interface. This can be achieved by writing overloaded constructors and methods and is covered later in this chapter. Polymorphism can also be achieved by having subclasses override superclass methods.
Let's take a simple look at this using Java code:
- First, we create a Vehicle class that, by default, extends Java's Object class.
- Next, we create a TwoWheeled class that extends the Vehicle class.
- Then, we create a Bicycle class that extends the TwoWheeled class.
- Lastly, we create a Driver class that creates an instance of the Bicycle class and runs four checks using the instanceof keyword:
public class Vehicle {
}
public class TwoWheeled extends Vehicle {
}
public class Bicycle extends TwoWheeled {
}
public class Driver {
public static void main(String[] args) {
Bicycle myBike = new Bicycle();
System.out.println("\nmyBike \"Instance of\" Checks");
if (myBike instanceof Bicycle)
System.out.println("Instance of Bicycle: True");
else
System.out.println("Instance of Bicycle: False");
if (myBike instanceof TwoWheeled)
System.out.println("Instance of TwoWheeled: True");
else
System.out.println("Instance of TwoWheeled: False");
if (myBike instanceof Vehicle)
System.out.println("Instance of Vehicle: True");
else
System.out.println("Instance of Vehicle: False");
if (myBike instanceof Object)
System.out.println("Instance of Object: True");
else
System.out.println("Instance of Object: False");
}
}
The output is as follows:

Driver class output
As you can see in the preceding screenshot, an instance of the Bicycle class is also an instance of TwoWheeled, Vehicle, and Object. This makes the myBike object polymorphic.