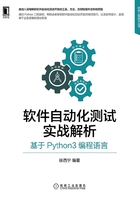
上QQ阅读APP看书,第一时间看更新
2.7.3 更多常见操作
针对字符串,Python设计了一系列的方法,让我们可以轻松应对常见的应用场景。
1)字符串内容的替换。
>>> plan = '2019年度计划' >>> plan.replace('2019', '2020') '2020年度计划'
2)获取字符串的长度。
>>> phone = '13800000831' >>> len(phone) 11
3)统计子字符串出现的频率。
>>> phone = '+86138000000831' >>> phone.count('8') 3 >>> lyrics = '说是就是不是也是,说不是就不是是也不是' >>> lyrics.count('是') 8 >>> lyrics.count('不是') 4
4)查找。字符串的查找有几种方式,最简单的一种是用in关键字。
>>> titles = 'Queen Daenerys Stormborn of the House Targaryen, the First of Her Name, Queen of the Andals, the Rhoynar and the First Men, The rightful Queen of the Seven Kingdoms and Protector of the Realm, Queen of Dragonstone, Queen of Meereen, Khaleesi of the Great Grass Sea, the Unburnt, Breaker of Chains and Mother of Dragons,regent of the realm' >>> 'Dragon' in titles True >>> 'Dinosaur' in titles False >>> 'Dinosaur' not in titles True
用in关键字来做字符串的查找很方便,但是不够强大。如果想要更多的查找选项,可以用index方法。index方法返回指定下标范围内找到的第一个子字符串的位置,如果不指定下标范围,则默认为整体搜索。
>>> titles.index('Queen') 0 >>> titles.index('Queen', 30) 72
使用index方法有几个值得注意的地方:
·它只会返回第一个被找到的子字符串的位置。
·可以不指定搜索范围,默认为整体搜索。
·可以只指定搜索范围的起始下标,在这种情况下,结束下标默认为字符串最后一个字符串的下标。
值得注意的一点是,如果没有找到指定的子字符串,index方法会抛出异常。
>>> titles.index('Peppa') Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: substring not found
index方法在没有找到子字符串的情况下会抛出异常,这个设计对程序员很不友好,因为很多时候我们只是想知道是不是存在指定的子串。
幸好,我们还有find方法。find方法和index方法的调用参数是一样的,如果查找成功,它们都会返回子字符串的位置。唯一的差别就是,如果查找失败,index会抛出异常,而find会返回–1。
要特别注意的是,find返回–1是表示查找失败,不是找到的子字符串的位置,而–1在Python里面也是一个合法的位置下标,这一点请一定要分清楚。
5)字符串以什么开头,又以什么结尾?
>>> 'Facebook'.startswith('Face') True >>> 'Facebook'.startswith('face') False >>> 'FACEBOOK'.startswith('FACE') True >>> 'FACEBOOK'.startswith('Face') False >>> 'Huawei'.endswith('wei') True
6)全部转成大写,或者只是首字母大写。
>>> 'facebook'.capitalize() 'Facebook' >>> 'FACeBOok'.capitalize() 'Facebook' >>> 'HuAwEi'.upper() 'HUAWEI' >>> 'HuAwEi'.lower() 'huawei'
7)分割字符串,用split。
>>> 'I see trees of green,red roses too,I seem them bloom,for me and you'. split(',') ['I see trees of green', 'red roses too', 'I seem them bloom', 'for me and you'] >>> 'I see trees of green,red roses too,I seem them bloom,for me and you'.split() ['I', 'see', 'trees', 'of', 'green,red', 'roses', 'too,I', 'seem', 'them', 'bloom,for', 'me', 'and', 'you']
8)去除前后空白字符,用strip,这里的l和r分别对应的是left和right。
>>> ' ok...\t '.lstrip() 'ok...\t ' >>> ' ok...\t '.rstrip() ' ok...' >>> ' ok...\t '.strip() 'ok...'
可以看到,字符串中的\t也被认为是空白字符,因为它是转义字符,代表一个tab。更多关于转义字符的知识,将会在后续章节中讲到。