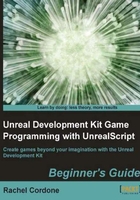
上QQ阅读APP看书,第一时间看更新
Time for action – Math!
- As an example, take a look at this code.
var float Float1, Float2; var int Int1; function PostBeginPlay() { Float2 = Int1 / Float1; 'log("Float2:" @ Float2); } defaultproperties { Int1=5 Float1=2.0 }
- We can divide an int by a float or vice versa, and we get the result we expect:
[0008.10] ScriptLog: Float2: 2.5000
However, if we divide an int by an int and assign it to a float, what would we expect the result to be?
- Let's take a look at this code:
var float Float1; var int Int1, Int2; function PostBeginPlay() { Float1 = Int1 / Int2; 'log("Float1:" @ Float1); } defaultproperties { Int1=5 Int2=2 }
With that it looks like we'd expect the same result. Let's take a look at the log:
[0007.66] ScriptLog: Float1: 2.0000
When dividing ints, the truncating happens before assigning the result, even if it's a float. Depending on what we're doing this may be what we want, but it's good to keep that in mind.
- Two other operators that can be used for simple math are increment (
++
) and decrement (–
).Int1 = 5; Int1++; 'log("Int1" @ Int1);
This would give us 6 in the log.
- For vectors and rotators, the arithmetic works with each element of the struct individually. For example, with the following code:
var vector Vect1, Vect2, VectResult; function PostBeginPlay() { VectResult = Vect1 + Vect2; 'log("VectResult:" @ VectResult); } defaultproperties { Vect1=(X=1.0,Y=4.5,Z=12.0) Vect2=(X=2.0,Y=4.0,Z=8.0) }
We get the following result in the log:
[0007.74] ScriptLog: VectResult: 3.00,8.5,20.00
As we can see, each individual element has been worked with separately. X added to X, Y to Y, and Z to Z.
- Vectors can also be multiplied or divided by floats and ints. This has the effect of changing the vector's VSize while keeping the direction the same.
What just happened?
The basic arithmetic operators are simple stuff, but when working with different types of variables it's important to remember how they'll respond to the operators.