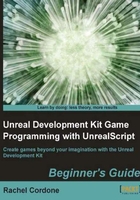
上QQ阅读APP看书,第一时间看更新
Time for action – Using floats
Floats are used when we need something that doesn't have nice neat values, like how far away something is or how accurate a weapon is. They're declared the same way as our bools and ints, so let's make one now.
- Replace our int declaration with this:
var float DistanceToGo;
- Floats have a default value of 0.0. Let's change our
PostBeginPlay
function to check this.function PostBeginPlay() { 'log("Distance to go:" @ DistanceToGo); }
- Compile and test, and our log should look like this:
[0007.61] ScriptLog: Distance to go: 0.0000
- We can see that unlike ints, floats will log with a decimal place. Let's see if we can change the value. Add this line to the beginning of our
PostBeginPlay
function:DistanceToGo = 0.123;
- Compile and test, and we should see the fraction show up in the log:
[0007.68] ScriptLog: Distance to go: 0.123
- Let's see what happens when we use the same line we did for our int. Change the line to this:
DistanceToGo = 10 / 3;
- Compile and test, and our log should look like this:
[0007.68] ScriptLog: Distance to go: 3.3333
What just happened?
Floats are used when we need precision in our numbers, such as calculating the distance between two points or the time remaining in a game. We also use them for complex math since they can have fractions.
Strings
No, these are not strings for our kittens to play with. In programming, strings store a series of characters, be it letters, numbers, symbols, or a combination of them. We can use them to hold the name of our character, messages to display on the screen, or the name of the weapon we're holding. Let's take a look at how to use them.