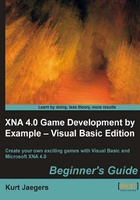
Time for action – fading pieces
- Add a new class to the Flood Control project called
FadingPiece
. - Add the following line to indicate that
FadingPiece
also inherits fromGamePiece
:Inherits GamePiece
- Add the following declarations to the
FadingPiece
class:Public AlphaLevel As Single = 1.0 Public Shared AlphaChangeRate As Single = 0.02
- Add a constructor for the
FadingPiece
class as follows:Public Sub New(type As String, suffix As String) MyBase.New(type, suffix) End Sub
- Add a method to update the piece:
Public Sub UpdatePiece() AlphaLevel = MathHelper.Max(0, AlphaLevel - AlphaChangeRate) End Sub
What just happened?
The simplest of our animated pieces, the FadingPiece
only requires an alpha value (which always starts at 1.0f
, or fully opaque) and a rate of change. The FadingPiece
constructor simply passes the parameters along to the base constructor.
When a FadingPiece
is updated, alphaLevel
is reduced by alphaChangeRate
, making the piece more transparent.
Managing animated pieces
Now that we can create animated pieces, it will be the responsibility of the GameBoard
class to keep track of them. In order to do that, we will define a Dictionary
object for each type of piece.
A Dictionary
is a collection object similar to a List
, except that instead of being organized by an index number, a Dictionary
consists of a set of key and value pairs. In an array or a List
, you might access an entity by referencing its index as in dataValues(2) = 12
. With a Dictionary
, the index is replaced with your desired key type. Most commonly, this will be a string value. This way, you can do something like fruitColors("Apple")="red"
.