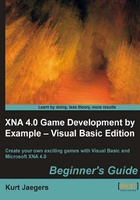
Time for action – making the connection
- Add the
PropagateWater()
method to theGameBoard
class:Public Sub PropagateWater(x As Integer, y As Integer, fromDirection As String) If (y >= 0) And (y <= GameBoardHeight) And (x >= 0) And (x <= GameBoardWidth) Then If boardSquares(x, y).HasConnector(fromDirection) And Not (boardSquares(x, y).PieceSuffix.Contains("W")) Then FillPiece(x, y) waterTracker.Add(New Vector2(x, y)) For Each pipeEnd As String In boardSquares(x, y).GetOtherEnds(fromDirection) Select Case pipeEnd Case "Left" PropagateWater(x - 1, y, "Right") Case "Right" PropagateWater(x + 1, y, "Left") Case "Top" PropagateWater(x, y - 1, "Bottom") Case "Bottom" PropagateWater(x, y + 1, "Top") End Select Next End If End If End Sub
- Add the
GetWaterChain()
method to theGameBoard
class:Public Function GetWaterChain(y As Integer) As List(Of Vector2) waterTracker.Clear() PropagateWater(0, y, "Left") Return waterTracker End Function
What just happened?
Together, GetWaterChain()
and PropagateWater()
are the keys to the entire Flood Control game, so understanding how they work is vital. When the game code wants to know if the player has completed a scoring row, it will call the GetWaterChain()
method once for each row on the game board:

The WaterTracker
list is cleared and GetWaterChain()
calls PropagateWater()
for the first square in the row, indicating that the water is coming from the Left
direction.
The PropagateWater()
method checks to make sure that the x
and y
coordinates passed to it exist within the board and, if they do, it checks to see if the piece at that location has a connector matching the fromDirection
parameter and that the piece is not already filled with water. If all of these conditions are met, that piece gets filled with water and added to the WaterTracker
list.
Finally, PropagateWater()
gets a list of all other directions that the piece contains (in other words, all directions the piece contains that do not match fromDirection
). For each of these directions PropagateWater()
recursively calls itself, passing in the new x
and y
location as well as the direction the water is coming from.