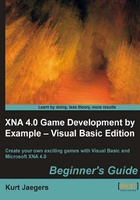
Time for action – GamePiece class methods – part 3 – connection methods
- Add the
GetOtherEnds()
method to theGamePiece
class:Public Function GetOtherEnds(startingEnd As String) As String() Dim Opposites As List(Of String) = New List(Of String)() For Each pipeEnd As String In _pieceType.Split(CChar(",")) If pipeEnd <> startingEnd Then Opposites.Add(pipeEnd) End If Next Return Opposites.ToArray() End Function
- Add the
HasConnector()
method to theGamePiece
class:Public Function HasConnector(direction As String) As Boolean Return _pieceType.Contains(direction) End Function
What just happened?
The GetOtherEnds()
method creates an empty List
object for holding the ends we want to return to the calling code. It then uses the Split()
method of the String
class to get each end listed in the _pieceType
. For example, the Top,Bottom
piece will return an array with two elements. The first element will contain Top
, and the second will contain Bottom
. The comma delimiter will not be returned with either string.
If the end in question is not the same as the startingEnd
parameter that was passed to the method, it is added to the list. After all of the items in the string have been examined, the list is converted to an array and returned to the calling code.
Tip
Type conversion
The Split()
method of the String
class accepts a Char
value, but if we simply supply the comma that we use within quotes (Split(",")
, for example), the result is itself a String
value. This would produce a compiler warning, informing us that we have an implicit conversion from string to char. We need to be careful that we are passing the right types of values to methods, so we are specifically casting the string to a char value using the CChar
built-in function. There are a number of these type-conversion functions in Visual Basic that support conversion to various data types, including a CType
method that accepts the type you wish to convert to as a parameter.
In the previous example, requesting GetOtherEnds("Top")
from a GamePiece
with a _pieceType
value of Top,Bottom
will return a string array with a single element containing Bottom
.
We will need this information in a few moments when we have to figure out which pipes are filled with water and which are empty.
The second function, HasConnector()
, simply returns true
if the _pieceType
string contains the string value passed in as the direction parameter. This will allow code outside the GamePiece
class to determine if the piece has a connector facing in any particular direction.