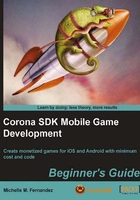
Time for action – scaling display objects on multiple devices
In our Display
Objects
project, we left off displaying a background image and three similar display objects in the simulator. When running the project on different devices, the coordinates and resolution size were most compatible with the iPhone only. When building applications for multiple devices across iOS and Android platforms, we can configure it using a config.lua
file that is compiled into the project and accessed at runtime. So let's get to it!
- In your text editor, create a new file and write out the following lines:
application = { content = { width = 320, height = 480, scale = "letterbox", xAlign = "left", yAlign = "top" }, }
- Save your script as
config.lua
in yourDisplay
Objects
project folder. - Mac users, launch your application in Corona under the iPhone device. Once you have done so, under the Corona Simulator menu bar, select Window | View As | iPhone 4. You will notice that the display objects fit perfectly on the screen and that there are no empty black spaces showing either.
- Windows users, launch your application in Corona under the Droid device. You will notice that all the content is scaled and aligned properly. Under the Corona Simulator menu bar, select Window | View As | NexusOne. Observe the similarities of content placement to that of the Droid. From left to right: iPhone 3G, iPhone 4, Droid, and NexusOne.
What just happened?
We have now learned a way to implement easy configuration to display our content across a variety of devices on iOS and Android. Content scaling features are useful for multiscreen development. If you look at the config.lua
file we created, the content width = 320
and height = 480
. This is the resolution size that the content is originally authored for. In this case, it is the iPhone 3G. Since we used scale = "letterbox"
, it enabled the content to uniformly scale up as much as possible, while still showing all content on the screen.
We also set xAlign = "left"
and yAlign = "top"
. This fills in the empty black screen space that shows on the Droid specifically. The content scaling is in the center by default so by aligning the content to the left and top of the screen the additional screen space will be taken away.
Dynamic resolution images
Earlier, we touched base with dynamic image resolution. iOS devices are a perfect example in this case. Corona has the capability to use base images (for devices on the 3GS and lower) and double-resolution images (for the iPhone 4 that has retina display) all within the same project file. Any of your double-resolution images can be swapped to your high-end iOS device without having to alter your code. This will allow your build to coincide with older devices and let you handle more complex multiscreen deployment cases. You will notice that dynamic image resolution works in conjunction with dynamic content scaling.
Using the line display.newImageRect( [parentGroup,] filename [, baseDirectory] w, h )
will call out your dynamic resolution images.
w
refers to the content width of the image and h
refers to the content height of the image.
For example:
myImage = display.newImageRect( "image.png", 128, 128 )
Remember that the two values represent the base image size, not the onscreen position of the image. You must define the base size in your code so that Corona knows how to render the higher-resolution alternative images. The contents of your project folder will be set up similarly as follows:
My New Project/ name of your project folder Icon.png required for iPhone/iPod/iPad Icon@2x.png required for iPhone/iPod with Retina display main.lua config.lua myImage.png Base image (Ex. Resolution 128 x 128 pixels) myImage@2x.png Double resolution image (Ex. Resolution 256 x 256 pixels)
When creating your double resolution image, make sure that it is twice the size of the base image. It's best when creating your display assets that you start with the double resolution image. Corona lets you select your own image naming patterns. The @2x
convention is one example that can be used but you have the option of naming suffixes to your personal preference. For now, we'll use the @2x
suffix since it distinguishes the double resolution reference. When you create your double resolution image, name it with the @2x
suffix included. Take the same image and resize it to 50 percent of the original size and then use the same filename without the @2x
included.
Other examples on naming suffixes can be:
@2
-2
-two
As mentioned earlier in the chapter, you have to define your image suffix for your double-resolution images in the imageSuffix
table in your config.lua
file. The content scale you set will allow Corona to determine the ratio between the current screen and base content dimensions. The following example uses the suffix @2x
to define double-resolution images:
application = { content = { width = 320, height = 480, scale = "letterbox", imageSuffix = { ["@2x"] = 2, }, }, }