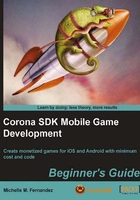
Time for action – placing images on screen
We're finally getting into the visually appealing part of this chapter by starting to add in display objects using images. We don't have to refer to the terminal window for now. So let's focus on the simulator screen. We'll begin by creating a background image and some art assets.
- First off, create a new project folder to your desktop and name it
Display Objects
. - In the
Chapter 2 Resources
folder, copy theglassbg.png
andmoon.png
image files into yourDisplay Objects
project folder. You can download the project files accompanying this book from the Packt website. - Launch your text editor and create a new
main.lua
file for your current project. - Write out the following lines of code:
local background = display.newImage( "glassbg.png", true ) local image01 = display.newImage( "moon.png", 110, 30 ) local image02 = display.newImage( "moon.png" ) image02.x = 160; image02.y = 200 image03 = display.newImage( "moon.png" ) image03.x = 160; image03.y = 320
The display object for your background variable should contain the filename of the background image in your project folder. For example, if the background image filename is called
glassbg.png
, then you would display the image as follows:local background = display.newImage( "glassbg.png", true )
Using
image02.x = 160; image02.y = 200
is the same as follows:image02.x = 160 image02.y = 200
The semicolon (
;
) indicates the end of a statement and is optional. It makes it easier to separate two or more statements in one line and saves adding extra lines to your code. - Save your script and launch your project in the simulator.
- You should see a background image and three other display objects that are the same as the image shown in as shown in the following screenshot. The display results will vary depending on which device you use to simulate.
The display objects for variables image01
, image02
, and image03
should contain the moon.png
filename. The filenames in your code are case-sensitive, so make sure that you write it exactly how it displays in your project folder.
What just happened?
Currently, background
is set to full resolution because we specified true
in the display object. We also have the image centered about its local origin since no top
or left
coordinates were applied.
When you observe the placement of image01
, image02
, and image03
in the simulator, they're practically in line with each other vertically, though the script style for image01
versus image02/image03
are written differently. This is because the coordinates for image01
are based on (left, top)
of the display object. You can optionally specify that the image's top-left corner be located at the coordinate (left, top)
; if you don't supply both coordinates, the image will be centered about its local origin.
Placement for image02
and image03
are specified from their local origin of the display object and positioned by local values of x and y properties of the device screen. The local origin is at the center of the image; the reference point is initialized at this point. Since we didn't apply (left, top)
values to image02
and image03
, further access to x or y properties are referred to the center of the image.
Now, you've probably noticed that the output from the iPhone 3G looks fine and dandy, but the output from the Droid shows that the display objects are not centered and the background image doesn't even fill the entire screen. We see that all the objects we specified are there but the scaling is off. That is because each iOS and Android device has different screen resolutions. The iPhone 3G has a screen resolution of 320 x 480 pixels and the Droid has a screen resolution of 480 x 854 pixels. What may look fine on one type of device may not look exactly the same on a different one. Don't worry, there is a simple solution to fix all that by using a config.lua
file that we will discuss in the next couple of sections.
Have a go hero – adjusting display object properties
Now that you know how to add images onto the device screen, try testing out the other display properties. Try doing any of the following:
- Change all the x and y coordinates of
image01
,image02
, andimage03
display objects - Choose any display object and change its rotation
- Change the visibility of a single display object
Refer to the display properties mentioned earlier in this chapter in case you're unsure how to do any of the preceding adjustments.