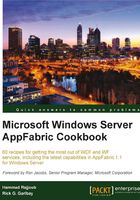
Initializing Cache Client using code
In the preceding chapter, we looked at the Client Libraries required for using Windows Server AppFabric's caching capabilities. Once these libraries are available, it is fairly straightforward to get started with common cache-related programming scenarios.
Windows Server AppFabric Caching Client needs connection settings to connect to a particular instance of a Cache Host. There are two ways a Cache Client can specify these settings:
- Code based: Using Windows Server AppFabric's Cache Client API to specify connection details programmatically
- Configuration based: Using the configuration file of a Cache Client to specify connection settings declaratively
In this recipe we will cover code-based configuration.
Getting ready
To be able to connect to Windows Server AppFabric Cache using the Client API, we need to ensure that the Cache Host we want to talk to is available. By using Windows Server AppFabric commandlets in PowerShell, we can identify and ensure that the particular instance is available for caching.
We will use the following AppFabric commands to check and see if the AppFabric Host is up and running.
You will need to launch AppFabric's Caching Administration Windows PowerShell tool with Admin privileges and run the following commands:
Use-CacheCluster:
To set the context for PowerShell.Start-CacheHost:
To start the cache host (if the cache host is already up and running, then we can skip this command), ORStart-CacheCluster:
To start the cache cluster. If you have only one node (for example, in Development environment) this is the same asStart-CacheHost
.Get-Cache:
To see if there is any cache defined on this host.

How to do it...
We will use Visual Studio 2010 to write programs against the AppFabric Caching API. Before we actually initialize the cache using the AppFabric API, we will quickly go through the following steps which will help us set up the development environment:
- Launch Visual Studio 2010 with administrative privileges.
- Start a new project using the C# Console Application project template and assign InitializeCacheWithCode as Solution name:
- Once the project is created, right-click on References and select Add Reference.
- Browse to
.Windows\System32\AppFabric
and add the following two assemblies:Microsoft.ApplicationServer.Caching.Core.dll
Microsoft.ApplicationServer.Caching.Client.dll
On a 64-bit OS, you may not be able to access the AppFabric folder directly using System32. However, AppFabric should still be available under
.\Windows\SysNative\AppFabric
. - Initialize the
DataCacheServerEndPoint
array with an instance that has servername = 'your-host'
andport='22233'
(or your own configured cache port):var cacheServerEndPoints = new[] { new DataCacheServerEndpoint("yourServerHost", 22233) };
Note
Your server host should be the cache Host Name as shown in the
Start-CacheCluster
command. You will also need to add the followingusing
statement to access AppFabric's caching related classes:using Microsoft.ApplicationServer.Caching
;. - Create an instance of
DataCacheFactoryConfiguration
and pass it an object ofDataCacheServerEndPoint:
var cacheConfiguration = new DataCacheFactoryConfiguration { Servers = cacheServerEndPoints };
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
- Create an instance of
DataCacheFactory
, passingDataCacheFactoryConfiguration
to the constructor:var cacheFactory = new DataCacheFactory(cacheConfiguration);
- Invoke
GetCache
onDataCacheFactory
by providing the name of the cache. The following code snippet shows a utility method showing the creation ofDataCache:
private static DataCache InitializeCache(string cacheName) { var cacheEndPoints = new[] { new DataCacheServerEndpoint("yourServerHost", 22233) }; var cacheConfiguration = new DataCacheFactoryConfiguration { Servers = cacheEndPoints }; var cacheFactory = new DataCacheFactory(cacheConfiguration); return cacheFactory.GetCache(cacheName); }
- The calling client can simply pass the name of the cache and the rest of the details around cache initialization can be handled by the method just defined:
DataCache cache = InitializeCache("referenceData"); Debug.Assert(cache != null);
Note
This may seem like a complex way of initializing a cache instance, but this complexity is worth it because of the highly configurable nature of the Windows Server AppFabric Cache API.
How it works...
DataCacheFactory
is responsible for providing access to DataCache
instances. DataCacheFactory
requires a configuration object of type DataCacheFactoryConfiguration,
which in turn requires an instance of array of DataCacheServerEndPoint
. The following is a schematic representation of the composition of DataCacheFactory
with respect to DataCacheFactoryConfiguration
and DataCacheServerEndPoint(s):

To be able to access DataCache
, we will need to create an array of DataCacheServerEndPoints
. Initialize it and assign it to an instance of the DataCacheFactoryConfiguration
class. We can then pass the DataCacheFactoryConfiguraiton
instance to our DataCacheFactory
class so that it can read the configuration via server end points and get the appropriate cache instance.
Note
Instantiation of DataCacheFactory
is a compute/resource extensive operation. Think about establishing network connections with server end points to start with. Considering this, it is recommended that you minimize the instantiation of DataCacheFactory
objects. Ideally, it makes sense to have only one instance of DataCacheFactory
available throughout the application lifecycle. Use of the Singleton design pattern is highly recommended to control the access to, and lifecycle of, DataCacheFactory
objects.
See also
In this recipe, we saw the use of the object model to construct a DataCache instance. In the following recipe we will once again create a DataCache instance, but without interacting with the detailed object model.