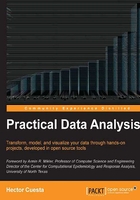
Interaction and animation
D3 provides a good support for interactions, transitions, and animations. In this example, we will focus on the basic way to add transitions and interactions to our visualization. This time we will use a very similar code to that of the bar chart example, in order to demonstrate how easy it is to add interactivity in visualization.
We need to define the font family and size for the labels and the style for the axis line.
<style> body { font: 14px arial; } .axis path, .axis line { fill: none; stroke: #000; } .bar { fill: gray; } </style>
Inside the body tag we need to refer to the library,
<body> <script src="http://d3js.org/d3.v3.min.js"></script> var formato = d3.format("0.0");
Now, we define the X and Y axis with a width of 1200
pixels and height 550
pixels.
var x = d3.scale.ordinal() .rangeRoundBands([0, 1200], .1); var y = d3.scale.linear() .range([550, 0]); var xAxis = d3.svg.axis() .scale(x) .orient("bottom"); var yAxis = d3.svg.axis() .scale(y) .orient("left") .tickFormat(formato);
Now, we select the element body
and create a new element <svg>
and define its size.
var svg = d3.select("body").append("svg") .attr("width", 1200) .attr("height", 550) .append("g") .attr("transform", "translate(20,50)");
Then, we need to open the TSV file sumPokemons.tsv
and read the values from the file and create the variable data
with two attributes type
and amount
.
d3.tsv("sumPokemons.tsv", function(error, data) { data.forEach(function(d) { d.amount = +d.amount; });
With the function map
we get our categorical values (type of pokemon) for the horizontal axis (x.domain
) and in the vertical axis (y.domain
) set our value axis with the maximum value by type (in case there is a duplicate value).
x.domain(data.map(function(d) { return d.type; })); y.domain([0, d3.max(data, function(d) { return d.amount; })]);
Now, we will create an SVG Group Element which is used to group SVG elements together with the tag <g>
. Then we use the transform
function to define a new coordinate system for a set of SVG elements by applying a transformation to each coordinate specified in this set of SVG elements.
svg.append("g") .attr("class", "x axis") .attr("transform", "translate(0," + height + ")") .call(xAxis); svg.append("g") .attr("class", "y axis") .call(yAxis)
Now, we need to generate .bar
elements and add them to svg
; then with the data(data)
function, for each value in data we will call the .enter()
function and add a rect
element. D3 allows selecting groups of elements for manipulation through the selectAll
function.
We want to highlight any bar just by clicking on it. First, we need to define a click event with .on('click',function)
. Next we will define the change of style for the bar highlighted with .style('fill','red');
. In the following figure we can see the highlighted bars Bug, Fire, Ghost, and Grass. Finally, we are going to set a simple animation using a transition transition().delay
with a delay between the appearing of each bar. (See the next bar chart).
Tip
For a complete reference about Selections follow the link https://github.com/mbostock/d3/wiki/Selections/.
svg.selectAll(".bar") .data(data) .enter().append("rect") .on('click', function(d,i) { d3.select(this).style('fill','red'); }) .attr("class", "bar") .attr("x", function(d) { return x(d.type); })
Tip
For a complete reference about Transitions follow the link https://github.com/mbostock/d3/wiki/Transitions/.
.attr("width", x.rangeBand()) .transition().delay(function (d,i){ return i * 300;}) .duration(300) .attr("y", function(d) { return y(d.amount); }) .attr("height", function(d) { return 550 - y(d.amount);}) ; }); // close the block d3.tsv
Tip
All the codes and datasets of this chapter can be found in the author GitHub repository at the link https://github.com/hmcuesta/PDA_Book/tree/master/Chapter3.
In the following figure we can see the result of the visualization.
