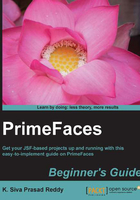
Time for action – passing data from the search users dialog to the source page
Let us add a new column Select
to the DataTable
in the searchUsers.xhtml
page to select a user, and pass the selected user's details back to source page. Perform the following steps:
- Create a
DataTable
component with aSelect
column to choose a user row using the following code:<p:dataTable id="usersTbl" value="#{userController.searchUsers}" var="user"> <p:column headerText="EmailId"> #{user.emailId} </p:column> <p:column headerText="Name"> #{user.firstName} #{user.lastName} </p:column> <p:column headerText="Select"> <p:commandButton icon="ui-icon-search" actionListener="#{userController.selectSearchUser(user)}" /> </p:column> </p:dataTable>
- When the user selects a row (user), close the dialog by passing the selected
user
object as data:public void selectSearchUser(User user){ RequestContext.getCurrentInstance().closeDialog(user); }
- Register the
dialogReturn
AJAX listener to the Search Users button, which will receive theSelectEvent
object as a parameter. We can obtain the selected user object fromSelectEvent
withevent.getObject()
, and update the UserDetailsPanelGrid
to display the selected user details:<p:commandButton value="Search Users" actionListener="#{userController.searchUsersForm}"> <p:ajax event="dialogReturn" listener="#{userController.handleSelectSearchUser}" update="UserDetails"/> </p:commandButton> <h:panelGrid id="UserDetails" columns="2"> <h:outputLabel value="EmailId" /> <h:outputText value="#{userController.searchUser.emailId}" /> <h:outputLabel value="FirstName" /> <h:outputText value="#{userController.searchUser.firstName}" /> </h:panelGrid> public void handleSelectSearchUser(SelectEvent event){ this.searchUser = (User) event.getObject(); }
What just happened?
We have created a CommandButton
button to open a dialog with the Search Users form. When the user searches for users, the results will be populated in a data table. When you select any user by clicking on the Select button, the selected user object will be passed back to the source page and display the results in the User Details panel grid.

Displaying FacesMessage in dialog
The dialog framework also provides a simple utility method, RequestContext.showMessageInDialog(FacesMessage)
to display FacesMessage
in a dialog. This comes in very handy when displaying the user action status once the application logic is executed:
<p:commandButton value="Delete" actionListener="#{userController.deleteUser}" /> public void deleteUser() { //logic to delete user RequestContext.getCurrentInstance().showMessageInDialog(new FacesMessage("User deleted successfully")); }
- At the moment,
<p:commandButton>
and<p:commandLink>
supportsdialogReturn
- Nested dialogs are not supported
- Calls to the
DialogFramework
API within a non-AJAX are ignored