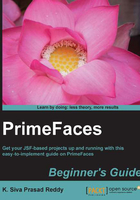
Time for action – displaying a dialog
Let us see how to create a basic Dialog component and show/hide based on button click events by performing the following steps:
- Create a form with a Dialog component and two buttons to show and hide the dialog using the following code:
<h:form> <p:commandButton value="ShowDialog" onclick="dlg1.show();" type="button" /> <p:commandButton value="HideDialog" onclick="dlg1.hide();" type="button" /> <p:dialog id="simpleDialog" header="Simple Dialog" widgetVar="dlg1" width="300" height="50"> <h:outputText value="PrimeFaces Simple Dialog" /> </p:dialog> </h:form>
What just happened?
We have created a Dialog component using <p:dialog>
and used two <p:commandButton>
components to perform show
and hide
actions on the dialog box using client-side API calls dlg1.show()
and dlg1.hide()
.
The Dialog component has the following attributes, which can be used to customize its behavior:
widgetVar
: This specifies the n Name of the client-side widget.visible
: When enabled, the dialog is visible by default. The default isfalse
.header
: This is the text of the header.footer
: This is the text of the footer.modal
: It enables modality, default is false.draggable
: This specifies drag ability, default is true.resizable
: It specifies resizability, default is true.closable
: This defines if close icon should be displayed or not, default is true .position
: This defines where the dialog should be displayed. By default, dialog is positioned at the center of the viewport. We can change the location by setting the position value using the following ways:- Single string value representing the position within viewport, such as position= "center". Other possible values are left, right, top, and bottom.
- Comma - separated x and y co-ordinate values such as position="150, 450".
- Comma - separated position values such as position="right,top".
showEffect
: This specifies the effect to use when showing the dialog.hideEffect
: This specifies the effect to use when hiding the dialog.onShow
: This determines the client-side callback to execute when dialog is displayed.onHide
: This determines the client-side callback to execute when dialog is hidden.minimizable
: This determines whether a dialog is minimizable or not, default isfalse
.maximizable
: This determines whether a dialog is maximizable or not, default isfalse
.appendToBody
: This appends Dialog component as a child of the document body. Default isfalse
.closeOnEscape
: This defines whether dialog should close on the escape key,default isfalse
.dynamic
: This enables lazy loading of the content with AJAX, default isfalse
.focus
: This defines which component to have focus by default.
We can apply different styles of effects while displaying or hiding the dialog using the showEffect
and hideEffect
attributes:
<p:dialog id="simpleDialog" header="Simple Dialog" widgetVar="dlg1" width="300" height="50" showEffect="bounce" hideEffect="explode"> <h:outputText value="PrimeFaces Simple Dialog" /> </p:dialog>
Available options for showEffect
and hideEffect
are: blind
, bounce
, clip
, drop
, explode
, fade
, fold
,highlight
, puff
, pulsate
, scale
, shake
, size
, slide
, and transfer
.
Using the Dialog component's client-side callbacks
Let us see how we can hook-up client-side JavaScript functions as callbacks using the onShow
and onHide
attributes.