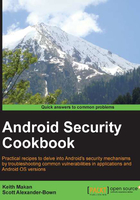
Signing Android applications
All Android applications are required to be signed before they are installed on an Android device. Eclipse and other IDEs pretty much handle application signing for you; but for you to truly understand how application signing works, you should try your hand at signing an application yourself using the tools in the Java JDK and Android SDK.
First, a little background on application signing. Android application signing is simply a repurposing of the JAR signing. It has been used for years to verify the authenticity of Java class file archives. Android's APK files aren't exactly like JAR files and include a little more metadata and resources than JAR files; so, the Android team needed to gear the JAR signing to suit the APK file's structure. They did this by making sure that the extra content included in an Android application forms part of the signing and verification process.
So, without giving away too much about application signing, let's grab an APK file and get it signed. Later in the walkthrough, we're going to try installing our "hand-signed" application on an Android device as an easy way to verify that we have in fact signed it properly.
Getting ready
Before we can begin, you will need to install the following things:
- Java JDK: This contains all of the necessary signing and verification tools
- APK file: This is a sample APK to sign
- WinZip: This is required for Windows machines
- Unzip: This is required for Ubuntu machines
Given that you may be using an APK file that's already signed, you will need to first remove the certificate and signature file from the APK file. To do this, you will need to perform the following steps:
- Unzip the APK file. It'd be waste to reiterate unpacking an APK file; so, if you need help with this step, refer to the Inspecting application certificates and signatures recipe.
- Once the APK file has been unzipped, you'll need to remove the
META-INF
folder. The Windows folks can simply open the unzippedAPK
folder and delete theMETA-INF
folder using the graphical user interface. This can be done from the command-line interface by executing the following command on Unix/Linux systems:rm –r [path-to-unzipped-apk]/META-INF
You should be ready to sign the application now.
How to do it...
Signing your Android application can be done by performing the following steps:
- You'll first need to set up a keystore for yourself because it will hold the private key that you will need to sign your applications with. If you already have a keystore set up, you can skip this step. To generate a brand new keystore on Windows and Unix/Linux distributions, you will need to execute the following command:
keytool –genkey –v -keystore [nameofkeystore] –alias [your_keyalias] –keyalg RSA –keysize 2048 –validity [numberofdays]
- After entering this command, keytool will help you set up a password for your keystore; you should make sure to enter something that you will actually remember! Also, if you at all intend to use this keystore for practical purposes, make sure to keep it in a very safe place!
- After you've set up the password for your keystore, keytool will begin prompting you for information that will be used to build your certificate; pay close attention to the information being requested and please answer as honestly as possible—even though this is not demonstrated in the following screenshot:
You should now have a brand new keystore set up with your new private key, public key, and self-signed certificate stored safely inside and encrypted for your protection.
- You can now use this brand new keystore to sign an application, and you can do that by executing the following command:
jarsigner –verbose –sigalg MD5withRSA –digestalg SHA1 –keystore [name of your keystore] [your .apk file] [your key alias]
- You'll be prompted for the password to the keystore. Once you enter it correctly,
jarsigner
will start signing the application in place. This means that it will modify the APK file that you gave it by adding theMETA-INF
folder with all of the certificate and signature-related details.And that's it. Signing an application is that easy. I've also inadvertently illustrated how to re-sign an application, namely, replace the signatures that were distributed with the application originally.
How it works...
To start off, let's have a look at the options supplied to keytool:
-genkey
: This option tells keytool that you'd like to generate some keys-v
: This option enables verbose output; however, this command is optional-keystore
: This option is used to locate the keystore you'd like to use to store your generated keys-alias
: This option is an alias for the key pair being generated-keyalg
: This option tells about the encryption algorithm used to generate the key; you can make use of either RSA or DSA-keysize
: This option specifies the actual bit length of the key you're going to generate-validity
: This option mentions the number of days for which the generated key will be valid; Android officially recommends using a value above 10,000 days
What keytool actually does with the public and private keys is store the public key wrapped inside an X.509 v3 certificate with it. This certificate is used to declare the identity of the public key holder and can be used to affirm that the mentioned public key belongs to the declared holder. This requires involvement from a trusted third party like a CA, but Android does not require public keys to be affirmed in this way. For more on how these certificates are used and structured, refer to the Inspecting application certificates and signatures recipe.
The options of jarsigner
are described in detail after the following command:
jarsigner –verbose –sigalg MD5withRSA –digestalg SHA1 –keystore [nameof your keystore] [your .apk file] [your key alias]
The following section explains the attributes of the preceding command:
-verbose
: This is used to enable verbose output-sigalg
: This is used to supply the algorithm to be used in the signing process-digestalg
: This is used to supply the algorithm that will compute the signatures for each of the resources in the.apk
file-keystore
: This is used to specify the keystore that you want to use[your .apk file]
: This is the.apk
file that you intend to sign[your key alias]
: This is the alias that you associated to the key/certificate pair
See also
- The Jarsigner documentation at http://docs.oracle.com/javase/6/docs/technotes/tools/windows/jarsigner.html
- The Signing your applications – Android Developer page at http://developer.android.com/tools/publishing/app-signing.html
- The Keytool documentation at http://docs.oracle.com/javase/6/docs/technotes/tools/solaris/keytool.html