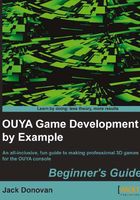
Time for action – creating and scripting 3D text
To demonstrate basic object properties in Unity, we'll create a 3D text object, which is a kind of game object that displays a string of text at a point in 3D space. The positions of all game objects are specified in X, Y, and Z coordinates, and each coordinate can be changed individually in the Inspector window. Now, perform the following steps:
- Open the Create menu and select 3D Text to create a New Text object.
- Use the Inspector window to position it at
0
,5
, and0
, and then set the Anchor dropdown to middle center and the Alignment dropdown to center.This puts your text directly in the center of the scene, elevated above the plane. The default text is Hello World, which is a common filler phase that developers enter when they are first testing text and display. This can be changed to any text you enter in the Inspector window under the Text Mesh pane.
Tip
Every object inspector window is an aggregation of all the object's components, which are organized into panes. Most objects share some components, such as Transform (position) and Mesh Renderer (appearance), but additional components are the ones that define an object's attributes and abilities.
Any displayed text in Unity can also be changed while the game is running by attaching a code script, which is what we'll do next. Make sure your scene is still positioned in the way we placed it previously, which should look as shown in the following screenshot through your Game window's main camera view:
Now is a good time to save your progress before we start writing code.
- Click on File and then Save Scene. The default save location will be your project's
Assets
folder, but it's a good practice to keep yourAssets
folder organized into subfolders, so create a new folder calledScenes
and save your scene within it as3dtext
. Your new folder will immediately appear inside your Project window below theAssets
folder, with your scene inside.Tip
Saving your scene isn't the same as saving your project; your project's saved files only store information about where different component files are stored (assets, scripts, and so on), while your scene's saved data tells the engine how everything is actually positioned within the scene. Think of your project files as useful information for your operating system, and your scene files as useful information for Unity.
Your scene is set up, saved, and ready for scripts, so we'll now begin coding by creating a C# script that controls the color of the text object.
- Right-click on your
Assets
folder and create another subfolder calledScripts
. - Right-click on the
Scripts
folder, move your mouse over Create, and select C# Script from the drop-down menu.By creating files from a folder's Create menu, they'll be created within that folder automatically.
- Name the script
ColorChanger
and double-click on it to open it in your code editor.Before we start adding code to the script, we'll do a quick explanation of the anatomy of a script, including all of the important keywords that you need to know and what they mean.
The first two lines of the script that begin with the
using
keyword denote the code libraries that this script relies on. Just below these is your script's class definition, which gives the code compiler basic information about the script.Tip
Keep in mind that your class name needs to match the script name in order for Unity to load it correctly. If you rename the script file from the Project window or manually in your filesystem in the future, the class name won't update automatically within the script, so make sure they match in order to avoid getting an error.
The
public
keyword means that other scripts can interact with this one, and theMonoBehavior
keyword is the name of the built-in script this one inherits basic properties from. The bracket after theinheritance
keyword and the bracket at the very end of the script encapsulate the entire class, and most of your code will be contained between these brackets. It is possible to write code for a class in a separate script if the situation calls for it, but you won't need to do that for the projects in this book.Every Unity script has two functions already defined in the code when you create it: the
Start
function and theUpdate
function. Functions are blocks of executable code that can be called to run by the game engine. TheStart
function is automatically called when an object with this script attached first enters the scene. TheUpdate
function is called for every frame, which is extremely quick (typically around 60 times per second), and it manages how the scripted object changes over the passage of time. There are several other built-in functions in Unity's API that can be extended in code by adding the function to a script, and you can create functions from scratch that execute your own code as well.Both of your starting functions have plain English descriptions written above them. The compiler doesn't interpret these descriptions, but they can be helpful for the programmer to keep notes; any text can be marked as a comment by inserting two forward slashes before it.
Notice that each of the pre-existing functions have parentheses and brackets directly after their names and the word void before them. The word before the function name represents the type of data that the function can return to; void means it won't be sending any data back to the caller. Other common return types include
int
(integer number),bool
(true or false), andstring
(readable text).The parentheses after the function name serve a purpose similar to the
return
keyword, but in the opposite direction; whatever is inside the parentheses denote data that must be sent to the function from the caller. The two pre-existing functions don't have anything inside the parentheses because they don't require data to be passed to them to function.Lastly, the brackets after the input parameter parentheses are the boundaries of the function. All code that the function contains goes between these two brackets, almost always separated by several lines. Right now, the brackets don't have anything inside of them, and that's what we're going to fill in now.
- Write a new line, as shown in the following code, with an explanatory comment in your
Start
function that will change the color of the text material to red:void Start () { //change the object's color to red gameObject.renderer.material.color = Color.red; }
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
The preceding line of code refers to
gameObject
, which represents the object that we attached the script to. It then points to renderer, a component of said object, and changes the rendered material's color to the standardColor.red
. Each line of non-commented code is terminated with a semicolon to tell the compiler to move on to the next line. Make sure each statement ends with a semicolon to avoid compiler errors.Tip
You're not expected to know these commands and keywords already; even if you've had experience with C#, coding in Unity is unique to the engine. You'll develop a natural memory of different code elements in Unity, but if you ever forget something, the Unity web documentation contains every function and keyword in the API.
Save your code in the File menu of your code editor to make sure all of your additions to the script were applied. The code will do what we want it to, but it still doesn't know which object we want to run it on; link the code file to your 3D text object by dragging it from your Project window onto the text object in your Hierarchy window. Alternatively, you can click on the Add Component button at the bottom of the item's Inspector window and find the
ColorChanger
script under the Scripts tab. If you select the text object, you'll see that the script is a newly added component in the Inspector window.Press the play arrow button (seen in the following screenshot) at the top of the Unity editor window to see your scene in action. The play button is useful because you can test right in the editor without having to deploy to the console. As soon as your game starts, your 3D text object will turn red according to the script we attached to it.
What just happened?
You're on the road to code! Every script in Unity is created from a basic template in the Project window, edited in a code editor, and linked to an object in your Hierarchy window by clicking-and-dragging. Scripts can be applied to any number of objects, but be careful that you don't attach scripts to objects that lack the attributes the code looks for. For instance, if you were to try to change the color of the camera, the engine would return an error, because the camera isn't a visible object, and thus doesn't have a renderer.
Tip
The names of functions and variables that you create are completely up to you, but many programmers follow a few common conventions to avoid confusion. In the Unity API, variables are camel case (first word all lowercase, first letter of all following words capitalized, as in mainPlayerPosition
). Unlike variables, every word in function names is usually capitalized, as in MovePlayerForward()
.
Have a go hero – flexing your new muscle
Now that you've got the basics down, play around with your new talent. A large part of programming and game development is finding out what works for you and what doesn't. Change small things at first as you start to learn the natural flow of coding, and make bigger changes if it starts to seem too easy. Here are some ideas to get you started:
- Explore the different available colors
- Attach your
ColorChanger
script to the plane object - Explore the different attributes that your editor's code hinting suggests
- Add a function to the script by copying the built-in function format
If you develop a taste for exploring on your own, look over some of the beginner topics in online question/answer forums on the web, such as www.stackoverflow.com.
You can also learn straight from the source by following Unity's beginner tutorials, found at http://unity3d.com/learn.