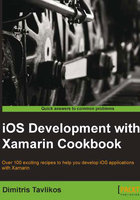
Using view controllers efficiently
iOS is very strict about memory usage. If an app uses too much memory, iOS will issue memory warnings. If we do not respond to these memory warnings accordingly by releasing resources that are not needed, it is very likely that iOS will terminate the app.
Getting ready
Let's see what we can do to avoid this situation. Create a new project in Xamarin Studio and name it EfficientControllerApp
.
How to do it…
Perform the following steps to complete this recipe:
- Add a view controller to the project and name it
MainController
. - Enter the following code in the
DidReceiveMemoryWarning
method of theMainController
class:Console.WriteLine("Main controller received memory warning!");
- Make the controller the root view controller of the app in
AppDelegate.cs
as follows:MainController mainController = new MainController(); window.RootViewController = mainController;
- Compile and run the app on the simulator.
- With iOS Simulator window active, navigate to Hardware | Simulate Memory Warning on the menu bar, as shown in the following screenshot:
- Check the Application Output pad in Xamarin Studio. You should see an output similar to the following:
2013-12-04 08:09:47.695 EfficientControllerApp[1383:80b] Received memory warning. 2013-12-04 08:09:47.709 EfficientControllerApp[1383:80b] Main controller received memory warning!
How it works...
This project does not provide any useful functionality. Its main purpose is to show how to get notified on memory warnings issued by iOS.
When a memory warning is issued, the DidReceiveMemoryWarning
method will be called on all instantiated view controllers that are currently in memory. When this method is called, we should make sure we release the resources that are not currently required. This way, we are making more memory available to the system.
iOS Simulator provides the option of simulating memory warnings so that we can test how our app will behave when memory is low. On a real device, we cannot force the system to issue memory warnings on demand. Note that although we can practically simulate an unlimited number of memory warnings on the simulator, the app will never be terminated. On the other hand, on the device, the app will be terminated after two or three memory warnings (the actual number varies according to memory usage), so we need to take this into account.
There's more...
View controllers are not the only object that can receive memory warnings. We can capture memory warning notifications by overriding the UIApplicationDelegate.ReceiveMemoryWarning(UIApplication)
method inside the AppDelegate
class, as follows:
public override void ReceiveMemoryWarning(UIApplication application) { //... }
See also
- The Creating a custom view controller recipe
- The Interface Builder recipe in Chapter 1, Development Tools