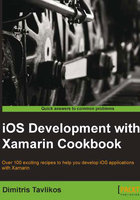
Modal view controllers
In this recipe, we will discuss how to display view controllers modally.
Getting ready
A modal view controller is any controller that is presented above other views or controllers. The concept is similar to displaying a Windows Form as a dialog, which takes control of the interface and does not allow access to other windows of the application unless it is dismissed. Create a new iPhone Empty Project in Xamarin Studio and name it ModalControllerApp
.
How to do it…
Perform the following steps:
- Add two view controllers to the project and name them
MainController
andModalController
. - Open the
MainController.xib
file in Interface Builder and add a button on its view with the titlePresent
. Create and connect the appropriate outlet for the button. - In the
MainController
class, add the following code in theViewDidLoad
method:this.buttonPresent.TouchUpInside += async (s, e) => { ModalController modalController = new ModalController(); await this.PresentViewControllerAsync(modalController, true); };
- Open the
ModalController.xib
file. Add a button on its view with the titleDismiss
and create the appropriate outlet for it. - Set its view background color to something other than white. Save the document and enter the following code in the
ViewDidLoad
method ofModalController
:this.buttonDismiss.TouchUpInside += async (s, e) => { await this.DismissViewControllerAsync (true); };
- Finally, add code to display the main controller in the
FinishedLaunching
method:MainController mainController = new MainController(); window.RootViewController = mainController;
- Compile and run the app on the simulator. Click on the Present button and watch the modal controller present itself on top of the main controller. Click on the Dismiss button to hide it.
How it works...
Each controller object has two methods that handle presenting and dismissing controllers modally. In our example, we call the PresentViewControllerAsync(UIViewController, bool)
method to present a controller, as follows:
this.buttonPresent.TouchUpInside += async (s, e) => { ModalController modal = new ModalController (); await this.PresentViewControllerAsync (modal, true); };
Its first parameter represents the controller we want to display modally, and the second parameter determines if we want the presentation to be animated. To dismiss the controller, we call its DismissViewControllerAsync(bool)
method, as follows:
await this.DismissViewControllerAsync (true);
It accepts only one parameter that toggles the animation for the dismissal.
In this example, we use async
/await
and the methods with the Async
suffix to present and dismiss a controller modally. These methods are included in Xamarin.iOS for convenience. We can also use PresentViewController
and DismissViewController
; both accept another parameter of the NSAction
type that represents the callback of the completion. However, no need to get into all that "trouble", right?
There's more...
We can define the transition style for a modal view controller presentation with the controller's ModalTransitionStyle
property. Enter the following line of code before presenting the modal controller:
modalController.ModalTransitionStyle = UIModalTransitionStyle.FlipHorizontal;
The main controller will flip to present the modal controller, giving the impression it is attached behind it.
Each controller that presents another controller modally provides access to its "child" controller through the ModalController
property. If you need to access the modal controller through this property, make sure to do it before the DismissViewControllerAsync
method is called.
In theory, we can present an unlimited number of modal controllers. Of course, there are two restrictions on this, which are as follows:
- Memory is not unlimited: View controllers consume memory, so the more view controllers we present, the worst performance we get.
- Bad user experience: Presenting many controllers modally might confuse the user.
In general, it is advised to not present more than one consecutive controller modally.
See also
- The Navigating through different view controllers and Providing controllers in tabs recipes