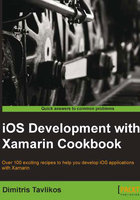
Creating a custom view
In this recipe, we will learn how to override the UIView
class and/or classes that derive from it to create custom views.
Getting ready
So far, we have discussed many of the available views to create iOS apps. There will be many cases, however, we will need to implement our own custom views. In this recipe, we will see how to create a custom view and use it.
Create a new iPhone Single View Application project in Xamarin Studio and name it CustomViewApp
.
How to do it...
The following are the steps to complete this recipe:
- Add a new C# class file in the project and name it
MyView
. - Implement it with the following code:
using System; using MonoTouch.UIKit; using MonoTouch.Foundation; using System.Drawing; namespace CustomViewApp { [Register("MyView")] public class MyView : UIView { private UILabel labelStatus; public MyView (IntPtr handle) : base(handle) { this.Initialize (); } public MyView (RectangleF frame) : base(frame) { this.Initialize (); } private void Initialize () { this.BackgroundColor = UIColor.LightGray; this.labelStatus = new UILabel (new RectangleF (0f, 400f, this.Frame.Width, 60f)); this.labelStatus.TextAlignment = UITextAlignment.Center; this.labelStatus.BackgroundColor = UIColor.DarkGray; this.AddSubview (this.labelStatus); } public override void TouchesMoved (NSSet touches, UIEventevtUIEvent evt) { base.TouchesMoved (touches, evt); UITouch touch = (UITouch)touches.AnyObject; PointF touchLocation = touch.LocationInView (this); this.labelStatus.Text = String.Format ("X: {0} - Y: {1}", touchLocation.X, touchLocation.Y); } } }
- Open the
CustomViewAppViewController.xib
file in Interface Builder and add aUIView
object on the main view. - Set its Class field in the Identity Inspector to
MyView
. - Save the document.
- Compile and run the app on the simulator. Tap and drag the view and watch the touch coordinates being displayed in the label at the bottom of the screen.
How it works...
The first thing to note when creating custom views is to derive them from the UIView
class and decorate them with the RegisterAttribute
, as shown in the following code:
[Register("MyView")] public class MyView : UIView
The RegisterAttribute
basically exposes our class to the Objective-C world. Note that the name we pass as its parameter must be the same name we enter in the Class field in the Identity Inspector. It is important to create the following constructor:
public MyView (IntPtr handle) : base(handle) {}
This constructor overrides the base class' UIView(IntPtr)
. This constructor is always being called when a view is initialized through the native code. If we do not override it, an exception will occur upon the initialization of the object. The other constructor that is used in this example is just provided as guidance on what might be used if the view was initialized programmatically:
public MyView (RectangleF frame) : base(frame) {}
Both these constructors call the Initialize()
method that performs the initialization we need, such as creating the label that will be used and setting the background colors.
Then, the TouchesMoved
method is overridden. This is the method that is executed when the user drags a finger on the view. Inside the method, we retrieve the UITouch
object from the method's NSSet parameter, using the following code:
UITouch touch = (UITouch)touches.AnyObject;
The UITouch
object contains information about user touches. We retrieve the touch's current location from the UITouch
object, using the following code:
PointF touchLocation = touch.LocationInView (this);
The UITouch
object's LocationInView
method accepts a parameter of the UIView
type, which declares in which view's coordinate system will the location be calculated. In this case, we are interested in the coordinates of MyView
.
There's more...
If we would like to initialize the custom view we created programmatically, we would enter the following code:
MyView myView = new MyView(new RectangleF(0f, 0f, 320f, 480f));
See also
- The Adding and customizing views recipe
- The Loading a view with a view controller recipe in Chapter 3, User Interface – View Controllers