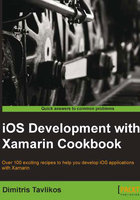
Displaying content larger than the screen
In this recipe, we will learn how to display content that extends beyond the screen's bounds.
Getting ready
In this recipe, we will discuss the UIScrollView
control. Create a new iPhone Single View Application project and name it ScrollApp
.
How to do it...
The following are the steps to create the project:
- Open the
ScrollAppViewController.xib
file in Interface Builder. - Add a
UIScrollView
object on its view and connect it to an outlet. Save the document. - Back in Xamarin Studio, add the following code in the
ScrollAppViewController
class:// Image view UIImageView imgView; public override void ViewDidLoad() { base.ViewDidLoad(); this.imgView = new UIImageView (UIImage.FromFile ("Kastoria.jpg")); this.scrollView.ContentSize = this.imgView.Image.Size; this.scrollView.ContentOffset = new PointF (200f, 50f); this.scrollView.PagingEnabled = true; this.scrollView.MinimumZoomScale = 0.25f; this.scrollView.MaximumZoomScale = 2f; this.scrollView.ViewForZoomingInScrollView = delegate(UIScrollView scroll) { return this.imgView; } ; this.scrollView.ZoomScale = 1f; this.scrollView.IndicatorStyle = UIScrollViewIndicatorStyle.White; this.scrollView.AddSubview (this.imgView); }
- Finally, add an image to the project and set its Build Action to BundleResource. An image larger than the screen size of 640 x 1136 pixels of iPhone 5S is preferable.
- Compile and run the app on the simulator. Tap and drag the image to display different portions. By pressing Alt + left-mouse click, you can simulate the pinch zooming function.
How it works...
The UIScrollView
is capable of managing content that expands beyond the screen size. The size of the content that the scroll view will display must be set in its ContentSize
property, as shown in the following code:
this.scrollView.ContentSize = this.imgView.Image.Size;
The ContentOffset
property shown in the following code defines the position of the content inside the scroll view's bounds:
this.scrollView.ContentOffset = new PointF (200f, 50f);
What this means is that the image's (x=200, y=50) point will be displayed at the origin (x=0, y=0) of UIScrollView
. To provide a zooming functionality for the content, we first set the MinimumZoomScale
and MaximumZoomScale
properties, as shown in the following code:
this.scrollView.MinimumZoomScale = 0.25f; this.scrollView.MaximumZoomScale = 2f;
The preceding code set the minimum and maximum zoom scale for the content. A value of 2
means that the content will be displayed double in size, while a value of 0.5
means that the content will be displayed at half its size.
For the actual zooming operation, we need to set the ViewForZoomingInScrollView
property, as shown in the following code:
this.scrollView.ViewForZoomingInScrollView = delegate(UIScrollView scroll) { return this.imgView; };
The ViewForZoomingInScrollView
property accepts a delegate
variable of the UIScrollViewGetZoomView
type and returns UIView
. Here, the image view that we created is returned, but another image view of a higher resolution can be used instead to provide better image quality when zooming. After the delegate
variable is assigned, the initial zoom scale is set using the following code:
this.scrollView.ZoomScale = 1f;
Finally, the scroll view's indicator style is set, as shown in the following code:
this.scrollView.IndicatorStyle = UIScrollViewIndicatorStyle.White;
Indicators are the two lines that appear when scrolling or zooming: one vertical line on the right side and one horizontal line on the bottom side of the scroll view. These lines inform the user of the position of the content.
There's more...
To provide a more pleasing scrolling and zooming effect to the user, the UIScrollView
exposes the Bounce
property. By default, it is set to true
, but we have the option to disable it by setting it to false
. Bouncing the content gives immediate feedback to the user that the bounds of the content have been reached, in either a horizontal or vertical direction. Furthermore, the AlwaysBounceHorizontal
and AlwaysBounceVertical
properties can be set individually. Setting one or both of these properties will make the scroll view bounce the content in the respective direction always, even if the content is equal to or smaller than the scroll view's bounds. Hence, no actual scrolling is needed.
The UIScrollView
class exposes some of the following very useful events:
Scrolled
: This occurs while the content is being scrolledDecelerationStarted
: This occurs when the user has started scrolling the contentDecelerationEnded
: This occurs when the user has finished scrolling, and the content has stopped moving
See also
- The Displaying images recipe
- The Displaying and editing text recipe
- The Navigating through the content divided into pages recipe