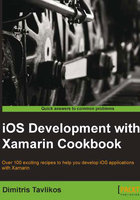
Displaying images
In this recipe, we will learn how to use the UIImageView
class to display images on screen.
Getting ready
In this recipe, we will see how to bundle and display images in a project. An image file will be needed for display. The image file used here is named Toroni.jpg
. Create a new iPhone Single View Application project in Xamarin Studio and name it ImageViewerApp
.
How to do it...
The following are the steps for this recipe:
- Open the
ImageViewerAppViewController.xib
file in Interface Builder. - Add a
UIImageView
object on its view. Connect theUIImageView
object with an outlet namedimageDisplay
. - Save the document.
- Back in Xamarin Studio, in the
ImageViewerAppViewController
class, enter the following code:public override ViewDidLoad() { base.ViewDidLoad(); this.imageDisplay.ContentMode = UIViewContentMode.ScaleAspectFit; this.imageDisplay.Image = UIImage.FromFile("Toroni.jpg"); }
- Right-click on the project in the Solution pad and navigate to Add | Add Files…. Select the image file you want to display and click on Open.
- Right-click on the image file you have just added and navigate to Build Action | BundleResource.
- Finally, compile and run the app on the simulator. The image you added to the project should be displayed on the screen, like in the following screenshot:
How it works...
The UIImageView
class is basically a view customized for displaying images. When you add an image in a project, its Build Action must be set to BundleResource in the Solution pad; otherwise, the image will not be copied into the app bundle. Fortunately, Xamarin Studio is smart enough to handle this setting automatically for images.
The ContentMode
property is very important when displaying images. It sets the way the UIView
(UIImageView
in this case) object will display the image. We have set it to UIViewContentMode.ScaleAspectFit
so that it will be resized to fit the area of UIImageView
, keeping the aspect ratio intact at the same time. If the ContentMode
property was left at its default ScaleToFill
value, the output would be something like the one shown in the following screenshot:

To set the image that UIImageView
should display, we set its Image
property with a UIImage
object, as shown in the following code:
this.imageDisplay.Image = UIImage.FromFile("Toroni.jpg");
The ContentMode
property accepts an enumeration type named UIViewContentMode
. The values provided are as follows:
ScaleToFill
: This is the default value of the baseUIView
object. It scales the content to fit the size of the view, changing the aspect ratio as necessary.ScaleAspectFit
: This scales the content to fit the size of the view, maintaining its aspect ratio. The remaining area of the view's content becomes transparent.ScaleAspectFill
: This scales the content to fill the size of the view, maintaining its aspect ratio. Some part of the content may be left out.Redraw
: When a view's bounds are changed, its content is not redrawn. This value causes the content to be redrawn. Drawing content is an expensive operation in terms of CPU cycles, so think twice before using this value with large content.Center
: This places the content at the center of the view, keeping its aspect ratio.Top
,Bottom
,Left
,Right
,TopLeft
,TopRight
,BottomLeft
, andBottomRight
: These align the content in the view with the corresponding value.
There's more...
The UIImage
class is the object that represents image information. The file formats it supports are listed in the following table:

Creating images for backgrounds provides developers with the ability to produce rich and elegant user interfaces for their apps. The preferred image file format for creating backgrounds for views is PNG. However, since iPhone 4 was released, the screen resolution was increased. To support both screen resolutions in an app, the iOS SDK provides an easy solution. Just save the image in the higher resolution and add a @2x
suffix to the file name just before the extension. For example, the name of a higher resolution version of a file named Default.png
would be Default@2x.png
. Also, no extra code is required to use both files. Just use the UIImage.FromBundle(string)
static method, passing the file name without an extension. The following line of code would load the appropriate file, depending on the screen resolution:
this.imageDisplay = UIImage.FromBundle("Default");
iOS takes care of loading the appropriate file, depending on the device the app is running on.
See also
- The Adding and customizing views recipe
- The Selecting images and videos recipe in Chapter 7, Multimedia Resources