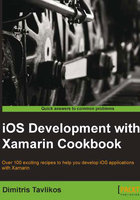
Adding and customizing views
In this recipe, we will discuss how to add and customize UIView
with Xcode's Interface Builder.
Getting ready
Adding views with Interface Builder is a simple task. Let's start by creating a new iPhone Single View Application
project in Xamarin Studio. Name the project FirstViewApp
and open the FirstViewAppViewController.xib
file with Interface Builder.
How to do it...
Perform the following steps:
- To add a view to the project, drag-and-drop a
UIView
object from the Library pad onto the main view. Make sure that it fits the entire window area. To makeUIView
accessible, create an outlet for it and name itsubView
.Note
The concept of outlets and how to use them is discussed in detail in Chapter 1, Development Tools.
- Select the view that we have just added and go to the Inspector pad. Select the Attributes tab, and select Dark Gray Color in the Background drop-down list. Now, select the Size tab and reduce the view's height by 60 points. Save the document.
- Compile and run the app on the simulator. The result should look like the one shown in the following screenshot:
The dark gray portion of the simulator's screen is the view that we have just added.
How it works...
We have successfully created an app that contains one view. Of course, this app does not provide any functionality. It is only meant to show how to add a view and display it.
Views are the essential components of an iOS app interface. Every visual user interface object inherits from the UIView
class. The concept is somewhat different from a form in .NET. A view manages content drawing, accepts other views as subviews, provides autosizing features, can accept touch events for itself and its subviews, and many of its properties can even be animated. Even UIWindow
inherits from UIView
. It is this class or its inheritors that iOS developers will use most frequently.
When a view that is added with Interface Builder is first instantiated at runtime, it sets its Frame
property with values that are set through the Inspector pad's Size tab. The Frame
property is of the RectangleF
type, and it defines the location of the view in its superview's coordinate system (in our case, the main window) and its size in points.
Note
In Objective-C, the frame
property of UIView is of the CGRect
type. This type has not been bound in Xamarin.iOS, and the more familiar System.Drawing.RectangleF
was used instead.
A superview is a view's parent view, while subviews are its child views. Views that have the same superview are described as siblings.
The default coordinate system in iOS originates from the top-left corner and extends towards the bottom and the right. The coordinate origin is always the same and cannot be changed programmatically.
The coordinate system of iOS is displayed in the following diagram:

When the Frame
property is set, it adjusts the Bounds
property. The Bounds
property defines the location of the view in its own coordinate system and its size in points. It is also of the RectangleF
type. The default location for the Bounds
property is (0,0), and its size is always the same as the view's Frame
value. Both these properties' sizes are connected to each other, so when you change the size of Frame
, the size of Bounds
changes accordingly and vice versa. You can change the Bounds
property to display different parts of the view.
A view's frame can exceed the screen in both location and position. That is, a view's frame with values (x = -50, y = -50, width = 1500, height = 1500) is perfectly acceptable, although it will not be completely visible on the screen of an iPhone.
There's more...
Another thing to note is that the UIView
class inherits from the UIResponder
class. The UIResponder
class is responsible for responding to and handling events. When a view is added to a superview, it becomes part of its responder chain. The UIView
class exposes the properties and methods of UIResponder
, and the ones we are interested in describing for now are the following two:
- IsFirstResponder property: This returns a Boolean value indicating whether the view is the first responder. Basically, it indicates if the view has focus.
- ResignFirstResponder(): This causes the view to lose focus.
If we would like to add a view on our main view programmatically, we would use the following UIView.AddSubview(UIView)
method:
this.View.AddSubview(this.subView);
The AddSubview
method adds its parameter, which is of the UIView
type, to the list of the caller's subviews and sets its Superview
parameter to the caller. A view will not be displayed unless it is added to a parent view with the AddSubview
method. Also, if a view already has a superview and it is added to another view with its AddSubview
method, its Superview
is changed to that of the new caller. What this means is that a view can have only one superview at a time.
For removing a view from its superview programmatically, call its RemoveFromSuperview
method. Calling this method on a view that has no superview does nothing. Care must be taken when we want to reuse the view we want to remove. We must keep a reference to it, or it might be released.
Another important property of UIView
is ContentMode
. ContentMode
accepts values of the UIViewContentMode
enumeration type. This property sets how the UIView
will display its content, usually an image. The default value of this property is UIViewContentMode.ScaleToFill
. This scales the content to fit the exact view's size, stretching it if necessary. The available values of UIViewContentMode
are explained in detail in the Displaying Images recipe later in this chapter.