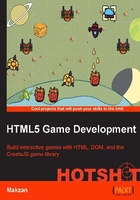
Creating a 3D card-flipping effect
In this task, we will take a look at how to create a 3D card-flipping effect. This effect will be used in our playing card element.
Prepare for lift off
We will need to prepare card graphics for this task. They are front-face.png
and back-face-pattern.png
.
Engage thrusters
We will start by defining the game elements in HTML, as we have done in the previous section:
- Inside
#game-scene
, we create two card elements with front and back faces:<div id="game-scene" class="scene out"> <div class="card a"> <div class="front face"></div> <div class="back face"></div> </div> <div class="card b flipped"> <div class="front face"></div> <div class="back face"></div> </div> </div>
- The core part in this section is the CSS styling. In the
game.css
file, we append the following styling to the card container for its front and back faces, flipped state, and the in-between transition:/* Card */ .card { width: 140px; height: 180px; perspective: 700; transition: all .3s ease-out; } /* card flipping related */ .card > .face { position: absolute; top: 0; left: 0; width: 100%; height: 100%; border-radius: 4px; backface-visibility: hidden; transition: all 0.3s ease-out; } .card > .face.front { background: #81d1e9 url(images/front-face.png); transform: rotate3d(0, 1, 0, 0deg); } .card > .face.back { background: #4474b5 url(images/back-face-pattern.png); transform: rotate3d(0, 1, 0, 180deg); border: 3px solid white; } .card.flipped > .face.front { transform: rotate3d(0, 1, 0, -180deg); } .card.flipped > .face.back { transform: rotate3d(0, 1, 0, 0deg); }
- The card-flipping effect requires only the HTML and CSS part. We use JavaScript just to trigger the flipped state. We define the following JavaScript in the
gameScene.onShow
function, which is called when the scene appears:gameScene.onShow = function() { setTimeout(function() { var cardA = document.querySelector('.card.a'); cardA.classList.add('flipped'); var cardB = document.querySelector('.card.b'); cardB.classList.remove('flipped'); }, 1000); };
Keep in mind that the JavaScript we just added to this task in step 3 is to test the card-flipping effect. We will be changing it in the next task.
Objective complete – mini debriefing
We have created a 3D card-flipping effect. Let's discuss how we built this effect.
3D transform
Transform applies position movement, rotation, and scaling to the elements. Moreover, it can transform in either a 2D or 3D space. The element is still 2D but it is put into a 3D space using the z-axis, as shown in the following figure:

The card-flipping effect
The DOM element is always a 2D pane with only one face. In reality, a card contains two faces—the front and back. So, in HTML, we create two faces inside each card element. We overlap them together and use the rotation transform to create the back and front faces.
The back face visibility is a key property to make the effect work. It hides the back face visibility when it is rotated to the back; or else, the rotated back face will still be there.
Another key property is the perspective. It is defined in the container of the 3D transformed elements. The higher a perspective value is, the more distortion it creates when it is rotated. The following figure shows how a card rotated in 45 degrees is displayed in different perspectives. A suitable perspective value creates a realistic 3D illusion. The distortion looks strange when the value is too low. The effect looks flat when the value is too high.

Toggling the flipping state
In this CSS-driven game, we use many CSS classes to act as a state. Take the flipping effect as an example; we add a "flipped" state to the card, and at the same time, the newly enabled CSS style defined in the .flipped
rules is applied to the card to create a flipping animation. It is actually more than just a styling. In the later tasks, we will query the cards with specific classes to perform actions, such as selecting the flipped player's cards or the selected player's card.
Also, the beauty of using separated classes such as card and flipped is the ability to extract common styles for each class category. In future tasks, we are going to have more classes applied to the card, including the player, opponent, and selected classes.
Classified intel
We used the y-axis to rotate the card. Another effect is rotating it on the x-axis. Furthermore, the rotation doesn't necessarily happen at the center point. The rotation origin can be set by the transform-origin property. The following two URLs link to examples that make use of 3D transform properties to create different effects:
The following screenshot shows the rotation effect:

Microsoft created an interactive tool to play with a combination of transform-related properties, which can be found at http://ie.microsoft.com/testdrive/graphics/hands-on-css3/hands-on_3d-transforms.htm.
David DaSandro wrote a detailed tutorial on how to make use of the 3D transform to create different effects, which can be found at http://desandro.github.io/3dtransforms/.