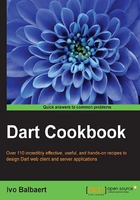
Using different settings in the checked and production modes
Often the development and deployment environments are different. For example, the app has to connect to a different database or a different mail server in either environment to use a mocked service in development and the real service in production, or something like that. How can we use different setups in both modes, or achieve a kind of precompiler directive-like functionality?
How to do it...
Perform the following steps to use different settings:
- Add a transformers section to
pubspec.yaml
with an environment line that specifies a map of the settings, names and values, as follows (see the code indev_prod_settings
):transformers: # or dev_transformers - $dart2js: environment: {PROD: "true", DB: "MongoPROD"}
- You can, for example, get the value of the DB setting from
const String.fromEnvironment('DB')
, as you can see in the following code:import 'dart:html'; void main() { print('PROD: ${const String.fromEnvironment('PROD')}'); bool prod = const String.fromEnvironment('PROD') == 'true'; if (prod) { // do production things window.alert("I am in Production!"); connectDB(const String.fromEnvironment('DB')); } else { // do developer / test things } log('In production, I do not exist'); } log(String msg) { if (const String.fromEnvironment('DEBUG') != null) { print('debug: $msg'); } } connectDB(String con) { // open a database connection }
- When run in the Dart VM (and in the checked mode), the console gives
PROD: null
as an output when run as JavaScript in Chrome; an alert dialog appears and the console in the Developer Tools shows PROD: true.
How it works...
The import initializer option requires a manual code change in order to switch the environment. The transformers option uses environment declarations that are provided by the surrounding system compiling or running the Dart program. This is better because it only requires changing the configuration file pubspec.yaml
. However, at the moment, it is only defined for dart2js, in order to deploy to JavaScript.
Make sure that the environment is indented; otherwise, you may get the error "transformers" must have a single key: the transformer identifier
.