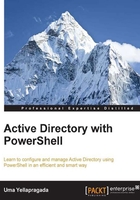
Managing user accounts
Active Directory is all about users and computers. Each user in the organization will have at least one account. There will be scenarios where a single user can have multiple accounts. This is very true in the case of IT users where one account is used for regular activities such as checking emails, browsing, and so on, whereas, the other privileged account is used for managing the infrastructure. Apart from this, there are service accounts that are designed to run a particular service. This shows how rapidly user accounts can grow in the Active Directory environment along with the necessity to manage them in a much more efficient way.
The following sections will explain how to perform user object operations using PowerShell.
Creating user accounts
Managing user accounts is one of the day-to-day jobs as a Windows administrator. New users join companies on a frequent basis and sometimes the volume might go high. In such cases, creating user accounts using conventional methods is time-consuming and prone to errors. So, relying on automation for creating new users would be a wise choice and less time-consuming.
In Active Directory, the manual account creation process involves Graphical User Interface (GUI) tools, such as Active Directory Users and Computers (ADUC) or Active Directory Administrative Center (ADAC).
Let's first take a look at how user creation can be done using ADAC.
ADAC was first introduced in Windows Server 2008 R2. It relies on Active Directory PowerShell cmdlets in Windows Server 2008 R2 and uses them in the background to perform the Active Directory operations. ADAC is further enhanced in Windows Server 2012 to expose the PowerShell commands that it uses in the background and repays study.
The AD module can be loaded into a normal PowerShell window using the following command:
Import-Module ActiveDirectory
Ensure that you open your PowerShell window in the elevated mode (run as Administrator) to gain maximum benefits from the module.
Now, let's see the user creation process using a GUI tool named ADAC, shown in the following screenshot:

There are two mandatory fields that must be provided in order to create a user account: Full Name and User SamAccountName. Other fields are optional at the time of user creation and can be updated later. You might have also noticed that the password is not specified at the time of creation, so Active Directory keeps this field in a disabled state until the password is set. Once the password is set by the administrator, the user object has to be enabled explicitly.
Similarly, when a user account is created using PowerShell, it has one mandatory property that must be passed, the Name
parameter. This parameter is equivalent to the Full Name value in UI. Also, the same
parameter value is used for the user's SamAccountName attribute at the time of user account creation using PowerShell.
A user account in Active Directory can be created using the New-ADUser
cmdlet. The following command is a small example to show how user account creation can be done:
New-ADUser -Name testuser1
When this command is executed from the PowerShell window, it creates a user account in the default user container. The account created will be in a disabled state because no password has been provided at the time of creation. This behavior is different when you create users using ADUC, where providing a password is mandatory.
The preceding one liner is just not sufficient for creating user accounts in the production environment. You are required to provide values for different attributes such as First Name, Last Name, Display Name, Password options (such as User must change password at next logon or not), Office address, phone numbers, Job title, Department, and the list goes on. So, we need to enhance our code to populate these properties at the time of login.
Before we start creating a full-fledged user account, let's see which properties can be populated by the New-ADUser
cmdlet at the time of user creation. You can get this simply by running the following help command:
Get-Help New-ADUser -Detailed
The Get-Help
cmdlet is the PowerShell cmdlet to see the help content of any other cmdlet. The usage of the –Detailed
switch tells the Get-Help
cmdlet to return all the help content for the given cmdlet. It includes a list of parameters, their syntax, an explanation of parameters, and examples.
To know more about each parameter, you can refer to the TechNet article at http://technet.microsoft.com/en-us/library/ee617253.aspx. This TechNet article explains about data types that each parameter stores and this is important to understand in order to read and write the attributes.
Tip
It is important to pay attention to the type of the value each parameter takes. If you provide any other data type apart from what it accepts, the New-ADUser
cmdlet ends in an error. The type of information that each parameter takes can be identified from the TechNet page at http://technet.microsoft.com/en-us/library/ee617253.aspx.
As you can see in the preceding command, there are various properties (called attributes in AD terminology) that you can set at the time of user creation. If the attribute you want to set is not present, then you can use the OtherAttributes
parameter to set it. Note that you need to provide other attribute names and values in hash table format while passing to the OtherAttributes
parameter. Don't worry about the use of hash tables. It is clearly explained later in this chapter in the Modifying user properties section.
Now, let's see how we can create a user account by passing all kinds of values that we want to set at the time of user creation. This example will cover some of the properties that are frequently used at the time of user object creation. However, you can modify this command and play around with setting other parameters. Practice makes one perfect!!!
The passthru
parameter is used to return the user object after creation of the account. If this parameter is not specified, the cmdlet will not show any output after successful creation of the object.
First, we need to prepare a password for the user to do the setting. Since the -AccountPassword
cmdlet requires the input to be in secure string format, we need to populate the $password
variable with the desired password, as shown by the following command:
$Password = Read-Host "Enter the password that you want to set" - AsSecureString
This will prompt you to enter the password and you will see asterisk symbols as you enter. Ensure that the password you enter should meet the password complexity of your domain, otherwise the following command will fail:
New-ADUser -Name Johnw -Surname "Williams" -GivenName "John" - EmailAddress "john.williams@techibee.ad" -SamAccountName "johnw" - AccountPassword $password -DisplayName "John Williams" -Department "Sales" -Country "US" -City "New York" -Path "OU=LAB,DC=Techibee,DC=AD" -Enabled $true -PassThru
Ensure that you update the -Path
parameter in the preceding command to reflect the distinguished name of the OU in your environment. Otherwise, the operation might fail.
Executing the preceding command from PowerShell will return the output shown in the following screenshot:

The output shows the path of the object where it is created and other properties we set during the creation process. By default, the output shows only a minimum set of attributes. You can see all current attributes and the values of a user object using the the Get-ADUser
cmdlet:
Get-ADUser -Identity JohnW -Properties *
Creating bulk user accounts
So far, we have seen how to create a single user account in Active Directory. Now, let's explore how to create multiple user accounts in one go.
The following command is sufficient enough if you want to create bulk user objects in the LAB environment without worrying about other properties such as department, email, and so on:
1..100 | foreach { New-ADUser -Name "Labuser$_" -AccountPassword $password -Path "OU=LAB,DC=techibee,DC=AD"}
It is a simple foreach loop that runs 100 times to create a user account with the name Labuser
suffixed by the number of iteration (such as Labuser1, Labuser2, and so on.) with the password set to the value of the $password
variable.
However, this is not sufficient to create user accounts in production environments. We need to populate several attributes at the time of creation. Ideally, system administrators receive the account creation information from HR in CSV format. So, the example being demonstrated in the following screenshot reads the account information from a CSV file, which has the details of user attributes, and creates user accounts based on this information.

Let's first read the content of the CSV file into a PowerShell variable. A cmdlet called Import-CSV
is available in PowerShell that can read the contents of the CSV file and return the output in object format. We can make use of it to read the contents, as shown in the following command:
$Users = Import-CSV <path of the saved CSV file> $Users | Format-Table
This command will read the contents of the CSV file into the $Users
variable. The next statement will show the contents of the variable in table format. It should look as follows:

Now, we have all user details in a variable, so let's proceed to create user accounts:
foreach($User in $Users) { New-ADUser -Name $User.LoginName -Surname $User.LastName - GivenName $User.FirstName -EmailAddress $User.Email - SamAccountName $User.LoginName -AccountPassword $Password - DisplayName $User.DisplayName -Country $User.Country -City $User.City -Path "OU=LAB,DC=Techibee,DC=AD" -Enabled $true - PassThru }
The preceding code will loop through each object in the $Users
variable and invoke creation of user accounts by passing the properties of the $User
object to parameters of the New-ADUser
cmdlet. The value of the -path
parameter in code has been hardcoded here, but you can make it part of the CSV file and pass it using the -Path
parameter during creation. Executing this code will create three accounts in Active Directory with the details given in the CSV file.
All you need to do is populate the CSV file with the details you want to apply to each user object and prepare the New-ADUser
cmdlet accordingly. Refer to Chapter 9, Miscellaneous Scripts and Resources for Further Learning for a more enhanced version of bulk user creation script.