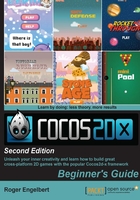
Cocos2d-x – an introduction
So what is a 2D framework? If I had to define it in as few words as possible, I'd say rectangles in a loop.
At the heart of Cocos2d-x, you find the Sprite
class and what that class does, in simple terms, is keep a reference to two very important rectangles. One is the image (or texture) rectangle, also called the source rectangle, and the other is the destination rectangle. If you want an image to appear in the center of the screen, you will use Sprite
. You will pass it the information of what and where that image source is and where on the screen you want it to appear.
There is not much that needs to be done to the first rectangle, the source one; but there is a lot that can be changed in the destination rectangle, including its position on the screen, its size, opacity, rotation, and so on.
Cocos2d-x will then take care of all the OpenGL drawing necessary to display your image where you want it and how you want it, and it will do so inside a render loop. Your code will most likely tap into that same loop to update its own logic.
Pretty much any 2D game you can think of can be built with Cocos2d-x with a few sprites and a loop.
Containers
Also important in Cocos2d-x is the notion of containers (or nodes). These are all the objects that can have sprites inside them (or other nodes.) This is extremely useful at times because by changing aspects of the container, you automatically change aspects of its children. Move the container and all its children will move with it. Rotate the container and well, you get the picture!
The containers are: Scene
, Layer
, and Sprite
. They all inherit from a base container class called node. Each container will have its peculiarities, but basically you will arrange them as follows:
Scene
: This will contain one or moreNode
, usuallyLayer
types. It is common to break applications into multiple scenes; for instance, one for the main menu, one for settings, and one for the actual game. Technically, each scene will behave as a separate entity in your application, almost as subapplications themselves, and you can run a series of transition effects when changing between scenes.Layer
: This will most likely containSprite
. There are a number of specializedLayer
objects aimed at saving you, the developer, some time in creating things such as menus for instance (Menu
), or a colored background (LayerColor
). You can have more than oneLayer
per scene, but good planning makes this usually unnecessary.Sprite
: This will contain your images and be added as children toLayer
derived containers. To my mind, this is the most important class in all of Cocos2d-x, so much so, that after your application initializes, when both aScene
and aLayer
object are created, you could build your entire game only with sprites and never use another container class in Cocos2d-x.Node
: This super class to all containers blurs the line between itself andLayer
, and evenSprite
at times. It has its own set of specialized subclasses (besides the ones mentioned earlier), such asMotionStreak
,ParallaxNode
, andSpriteBatchNode
, to name a few. It can, with a few adjustments, behave just asLayer
. But most of the time you will use it to create your own specialized nodes or as a general reference in polymorphism.
The Director and cache classes
After containers comes the all-knowing Director
and all-encompassing cache objects. The Director
object manages scenes and knows all about your application. You will make calls to it to get to that information and to change some of the things such as screen size, frame rate, scale factor, and so forth.
The caches are collector objects. The most important ones are TextureCache
, SpriteFrameCache
, and AnimationCache
. These are responsible for storing key information regarding those two important rectangles I mentioned about earlier. But every type of data that is used repeatedly in Cocos2d-x will be kept in some sort of cache list.
Both Director
and all cache objects are singletons. These are special sort of classes that are instantiated only once; and this one instance can be accessed by any other object.
The other stuff
After the basic containers, the caches and the Director
object, comes the remaining 90 percent of the framework. Among all this, you will find:
- Actions: Animations will be handled through these and what a treat they are!
- Particles: Particles systems for your delight.
- Specialized nodes: For things such as menus, progress bars, special effects, parallax effect, tile maps, and much, much more.
- The macros, structures, and helper methods: Hundreds of time-saving, magical bits of logic. You don't need to know them all, but chances are that you will be coding something that can be easily replaced by a macro or a helper method and feel incredibly silly when you find out about it later.
Do you know C++?
Don't worry, the C part is easy. The first plus goes by really fast, but that second plus, oh boy!
Remember, it is C. And if you have coded in Objective-C with the original Cocos2d, you know good old C already even if you saw it in between brackets most of the time.
But C++ also has classes, just like Objective-C, and these classes are declared in the interface files just like in Objective-C. So let's go over the creation of a C++ class.