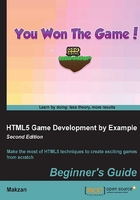
Time for action – flipping a card with CSS3
We are going to start a new project and create a card-flipping effect when we click on the playing card:
- Let's continue with our previous code example.
- The card now contains two faces: a front face and a back face. Replace the following code in the
body
tag in the HTML code:<section id="game"> <p id="cards"> <p class="card"> <p class="face front"></p> <p class="face back cardAK"></p> </p> <!-- .card --> <p class="card"> <p class="face front"></p> <p class="face back cardAQ"></p> </p> <!-- .card --> </p> <!-- #cards --> </section> <!-- #game --> <script src="js/jquery-2.1.3.min.js"></script>
- Then, create a new
css3flip.css
file in thecss
folder to test the flipping effect. - In the
index.html
file, change the CSS external link to thecss3flip.css
file:<link rel="stylesheet" href="css/css3flip.css" />
- Now, let's add the styles to the
css3flip.css
:#game { background: #9c9; padding: 5px; } /* Define the 3D perspective view and dimension of each card. */ .card { perspective: 600px; width: 80px; height: 120px; }
- There are two faces on each card. We are going to slowly rotate the face of the card. Therefore, we define how the face transitions by CSS3's
transition
property. We also hide the back face's visibility. We will look at the detail of this property later:.face { border-radius: 10px; width: 100%; height: 100%; position: absolute; transition: all .3s; backface-visibility: hidden; }
- Now, it is time to style each inpidual face. The front face has a higher z-index than the back face:
.front { background: #966; } .back { background: #eaa; transform: rotate3d(0,1,0,-180deg); }
- When we flip the card, we rotate the front face to the back and the back face to the front. We also swap the z-index of the front and back faces:
.card-flipped .front { transform: rotate3d(0,1,0,180deg); } .card-flipped .back { transform: rotate3d(0,1,0,0deg); } .cardAK { background: url(../images/AK.png); } .cardAQ { background: url(../images/AQ.png); }
- Next, we will add logic after loading the jQuery library to toggle the card-flipped status when we click on the card:
<script> (function($){ $(function(){ $("#cards").children().each(function(index) { // listen the click event on each card DIV element. $(this).click(function() { // add the class "card-flipped". // the browser will animate the styles // between current state and card-flipped state. $(this).toggleClass("card-flipped"); }); }); }); })(jQuery); </script>
- The styles and the scripts are now ready. Let's save all the files and preview it in our web browser. Click on the playing card to flip it over, and click on it again to flip it back.
What just happened?
We have created a card-flipping effect that can be toggled by a mouse click. You can try out the example at http://makzan.net/html5-games/simple-card-flip/.
The example made use of several CSS transform properties and JavaScript to handle the mouse click event.
Toggling a class with jQuery's toggleClass function
We apply the card-flipped
class to the card element when the mouse is clicked on the card. On the second click, we want to remove the applied card-flipped style so that the card flips back again. This is called toggling a class style.
jQuery provides us with a handy function named toggleClass
to add or remove classes automatically, depending on whether the class is applied or not.
To use the function, we simply pass the classes that we want to toggle as an argument.
For example, the following code adds or removes the card-flipped
class to an element with the ID as card1
:
$("#card1").toggleClass("card-flipped");
The toggleClass
function accepts the toggle instruction from more than one class at the same time. We can pass in several class names and separate them by using a space. Here is an example of toggling two classes at the same time:
$("#card1").toggleClass("card-flipped scale-up");
Introducing CSS' perspective property
CSS3 lets us present elements in 3D, and we have been able to transform the elements in the 3D space. The perspective
property defines how the 3D perspective view looks. You can treat the value as far as you are looking at the object. The closer you are, the more perspective distortion there is on the viewing object.
The following two 3D cubes demonstrate how different perspective values change the perspective view of the element:

Have a go hero
The cube is created by putting six faces together with 3D transforms applied to each face. It used the techniques that we've discussed. Try to create a cube and experiment with the perspective
property.
The following web page gives a comprehensive explanation on creating the CSS3 cube, and it also explains how to control the rotation of the cube with the keyboard:
http://paulrhayes.com/experiments/cube-3d/
Introducing backface-visibility
Before the backface-visibility
property was introduced, all the elements on the page presented their front face to the visitor. Actually, there was no concept of the front face or the back face of the element because presenting the front face was the only choice. While CSS3 introduces the rotation in three axes, we can rotate an element so that its face is on the back. Try looking at your palm and rotating your wrist, your palm turns and you see the back of your palm. This happens to the rotated elements too.
CSS3 introduces a property named backface-visibility
to define whether or not we can see the back face of the element. By default, it is visible. The following figure demonstrates the two different behaviors of the backface-visibility
property:

Note
You can read more detailed information about different properties and functions in CSS 3D transforms on its official Webkit blog at http://webkit.org/blog/386/3d-transforms/.