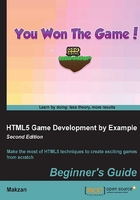
Time for action – moving a playing card around
In this example, we will place two playing cards on a web page and transform them to a different position, scale, and rotation. We will tween the transformation by setting the transition:
- To do this, create a new project folder with the following hierarchy. The
css3transition.css
andindex.html
files are empty now and we will add the code later. Thejquery-2.1.3.min.js
file is the jQuery library that we used in the previous chapter.index.html js/ js/jquery-2.1.3.js css/ css/css3transition.css images/
- We are using two playing card graphic images in this example. They are
AK.png
andAQ.png
. The images are available in the code bundle or you can download them from the book assets website at http://mak.la/book-assets/. - Put the two card images inside the
images
folder. - The next thing is to code the HTML with two card DIV elements. We will apply CSS transition style to these two cards elements when the page is loaded:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Getting Familiar with CSS3 Transition</title> <link rel="stylesheet" href="css/css3transition.css" /> </head> <body> <header> <h1>Getting Familiar with CSS3 Transition</h1> </header> <section id="game"> <p id="cards"> <p id="card1" class="card cardAK"></p> <p id="card2" class="card cardAQ"></p> </p> <!-- #cards --> </section> <!-- #game --> <footer> <p>This is an example of transitioning cards.</p> </footer> <script src="js/jquery-2.1.3.min.js"></script> <script> $(function(){ $("#card1").addClass("move-and-scale"); $("#card2").addClass("rotate-right"); }); </script> </body> </html>
- It is time to define the visual styles of the playing cards via CSS. This contains basic CSS 2.1 properties and CSS3's new properties. In the following code, the new CSS3 properties are highlighted:
body { background: LIGHTGREY; } /* defines styles for each card */ .card { width: 80px; height: 120px; margin: 20px; position: absolute; transition: all 1s linear; } /* set the card to corresponding playing card graphics */ .cardAK { background: url(../images/AK.png); } .cardAQ { background: url(../images/AQ.png); } /* rotate the applied DOM element 90 degree */ .rotate-right { transform: rotate3d(0, 0, 1, 90deg); } /* move and scale up the applied DOM element */ .move-and-scale { transform: translate3d(150px, 150px, 0) scale3d(1.5, 1.5, 1); }
- Let's save all the files and open the
index.html
file in the browser. The two cards should animate as shown in the following screenshot:
What just happened?
We just created two animation effects by using the CSS3 transition to tween the transform
property.
Here is the usage of CSS transform:
transform: transform-function1 transform-function2;
The arguments of the transform
property are functions. There are two sets of functions: the 2D and 3D transform functions. CSS transform functions are designed to move, scale, rotate, and skew the target DOM elements. The following sections show the usage of the transform functions.
2D transform functions
The 2D rotate
function rotates the element clockwise on a given positive argument and counter-clockwise on a given negative argument:
rotate(angle)
The translate
function moves the element by the given x and y displacement:
translate (tx, ty)
We can translate the x or y axis independently by calling the translateX
and translateY
function as follows:
translateX(number) translateY(number)
The scale
function scales the element by the given sx
and sy
vectors. If we only pass the first argument, then sy
will be of the same value as sx
:
scale(sx, sy)
In addition, we can independently scale the x and y axis as follows:
scaleX(number) scaleY(number)
3D transform functions
The 3D rotation function rotates the element in 3D space by the given [x, y, z] unit vector. For example, we can rotate the y axis 60 degrees by using rotate3d(0,
1,
0,
60deg)
:
rotate3d(x, y, z, angle)
We can also rotate one axis only by calling the following handy functions:
rotateX(angle) rotateY(angle) rotateZ(angle)
Similar to the 2D translate
function, translate3d
allows us to move the element in all the three axes:
translate3d(tx, ty, tz) translateX(tx) translateY(ty) translateZ(tz)
Also, the scale3d
scales the element in the 3D spaces:
scale3d(sx, sy, sz) scaleX(sx) scaleY(sy) scaleZ(sz)
The transform
functions that we just discussed are common, and we will use them many times. There are several other transform
functions that are not discussed; they are matrix
, skew
, and perspective
.
If you want to find the latest CSS Transforms working spec, you can visit the W3C website of CSS Transforms Modules at: http://www.w3.org/TR/css3-3d-transforms/.
Tweening the styles using CSS3 transition
There are tons of new features in CSS3. Transition module is one among them that affects us most in game designing.
What is CSS3 transition? W3C explains it in one sentence:
CSS transitions allows property changes in CSS values to occur smoothly over a specified duration.
Normally, when we change any properties of the element, the properties are updated to the new value immediately. Transition slows down the changing process. It creates smooth in-between easing from the old value towards the new value in the given duration.
Here is the usage of the transition
property:
transition: property_name duration timing_function delay
The following table explains each of the parameters used in the transition
property:

We can put several transition
properties in one line. For example, the following code transitions the opacity in 0.3 seconds and background color in 0.5 seconds:
transition: opacity 0.3s, background-color 0.5s
We can also define each transition property inpidually by using the following properties:
transition-property, transition-duration, transition-timing-function and transition-delay
Tip
Modules of CSS3
According to W3C, CSS3 is unlike CSS 2.1 in that there is only one CSS 2.1 spec. CSS3 is pided into different modules. Each module is reviewed inpidually. For example, there is a transition module, 2D/3D transforms module, and the Flexible Box Layout module.
The reason for piding the spec into modules is because the pace at which the work of each part of the CSS3 progresses is not the same. Some CSS3 features are rather stable, such as border radius, while some have not yet settled down. Dividing the whole spec into different parts allows the browser vendor to support modules that are stable. In this scenario, slow-paced features will not slow down the whole spec. The aim of the CSS3 spec is to standardize the most common visual usage in web designing and this module fits this aim.
Have a go hero
We have translated, scaled, and rotated the playing cards. How about we try to change different values in the example? There are three axes in the rotate3d
function. What will happen if we rotate the other axis? Experiment with the code yourself to get familiar with the transform and transition modules.