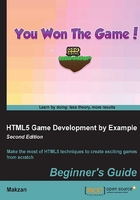
Time for action – installing the jQuery library
We will create our HTML5 Ping Pong game from scratch. It may sound as if we are going to be preparing all the things ourselves. Luckily, we can use a JavaScript library to help us. jQuery is the JavaScript library that is designed to navigate the DOM elements easily, manipulate them, handle events, and create an asynchronous remote call. We will be using this library in the book to manipulate DOM elements. It will help us to simplify our JavaScript logic:
- Create a new folder named
pingpong
as our project directory. - Inside the
pingpong
folder, we will create the following file structure, with three folders—js
,css
, andimages
—and anindex.html
file:index.html js/ js/pingpong.js css/ css/pingpong.css images/
- Now, it's time to download the jQuery library. Go to http://jquery.com/.
- Select Download jQuery and click on Download the compressed, production jQuery 2.1.3.
- Save
jquery-2.1.3.min.js
within thejs
folder that we created in step 2. - Open
index.html
in text editor and insert an empty HTML template:<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Ping Pong</title> <link rel="stylesheet" href="css/pingpong.css"> </head> <body> <header> <h1>Ping Pong</h1> </header> <p id="game"> <!-- game elements to be here --> </p> <footer> This is an example of creating a Ping Pong Game. </footer> <script src="js/jquery-2.1.3.min.js"></script> <script src="js/pingpong.js"></script></body> </html>
- Finally, we have to ensure that jQuery is loaded successfully. To do this, place the following code into the
js/pingpong.js
file:(function($){ $(function(){ // alert a message alert("Welcome to the Ping Pong battle."); }); })(jQuery);
- Save the
index.html
file and open it in the browser. You should see the following alert window showing our text. This means that our jQuery is correctly set up:
What just happened?
We just created a basic HTML5 page with jQuery and ensured that the jQuery is loaded correctly.
New HTML5 doctype
The DOCTYPE
and meta
tags are simplified in HTML5.
In HTML 4.01, we declare doctype using the following code:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
This is a long line of code, right? While in HTML5, the doctype declaration couldn't have been more simpler:
<!DOCTYPE html>
We do not even have the HTML version in the declaration. This is because HTML5 is now a living standard without a version number.
Header and footer
HTML5 comes with many new features and improvements, and one of them is semantics. HTML5 adds new elements to improve semantics. We just used two of the elements: header
and footer
. Header gives an introduction to the section or the entire page. Therefore, we put the h1
title inside the header. Footer, like the name suggests, contains the footer information of the section or the page.
Note
A semantic HTML means that the markup itself provides meaningful information to the content instead of only defining the visual outlook.
The best practice to place the JavaScript code
We put the JavaScript code right before the closing </body>
tag and after all the content in the page. There is a reason for putting the code there instead of putting it inside the <head></head>
section.
Normally, browsers load content and render them from top to bottom. If the JavaScript code is put in the head
section, then the content of the document will not be loaded until all the JavaScript code is loaded. Actually, all rendering and loading will be paused if the browsers load a JavaScript code in the middle of the page. This is the reason why we want to put the JavaScript code at the bottom, when possible. In this way, we can deliver the HTML content to our readers faster.
At the time of writing this book, the latest jQuery version is 2.1.3. This is why the jQuery file in our code examples is named jquery-2.1.3.min.js
. The version number in the filename ensures that web developers don't get confused with different versions of the same filename in different projects. This version number will be different, but the usage should be the same, unless there is a big change in jQuery without backward compatibility.
Note
Please note that a few JavaScript libraries have to put the <head>
tag before loading any HTML elements. When you're using third-party libraries, please check whether they have such a requirement.
Choosing the jQuery file
For the jQuery library, there are currently two major versions; they are 1.x and 2.x. The 1.x version keeps backward compatibility to older browsers, mainly for IE versions 6, 7, and 8. Since our HTML5 games target modern browsers, we chose the 2.x version that has dropped support to IE 8 or the older versions.
There are two common ways to include the jQuery library. We can either download a hosted version or use the CDN version. Hosted version means that we download the file, and we host the file ourselves. CDN stands for Content Delivery Network. The jQuery files are hosted in several central servers to improve the file downloading time. For the CDN version, we can find the URL at http://code.jquery.com. We can directly include the file with the <script>
tag in HTML as: <script src="http://code.jquery.com/jquery.min.js"></script>
.
Otherwise, we can specify the version number in the filename as: <script src="http://code.jquery.com/jquery-2.1.3.min.js"></script>
.
Running jQuery inside a scope
We need to ensure that the page is ready before our JavaScript code is executed. Otherwise, we may get an error when we try to access an element that is not yet loaded. jQuery provides us with a way to execute the code after the page is ready by using the following code:
jQuery(document).ready(function(){ // code here. });
Most of the time, we uses a $
sign to represent jQuery. This is a shortcut that makes calling our jQuery functions much easier. So essentially, we use the following code:
$(function(){ // code here. });
When we call $(something)
, we are actually calling jQuery(something)
.
There may be conflicts on the $
variables if we use multiple JavaScript libraries in one project. For best practice, we use an anonymous function to pass the jQuery object into the function scope where it becomes a $
sign:
(function($){ // jQuery code here with $. })(jQuery);
An anonymous function is a function definition that has no name. That's why it's called anonymous. Since we cannot refer to this function anymore, the anonymous function always executes itself. JavaScript's variable scope is bound to the function scope. We often use anonymous function to control certain variables' availability. For instance, we passed the jQuery into the function as the $
variable in our example.
Running our code after the page is ready
$(function_callback)
is another shortcut for the DOM elements' ready
event. The reason we need jQuery ready
function is to prevent the execution of JavaScript logic before the HTML DOM elements are loaded. Our function that is defined in the jQuery ready
function is executed after all the HTML elements are loaded.
It is identical to the following line of code:
$(document).ready(function_callback);
Note
Note that the jQuery ready
event fires after the HTML structure (DOM tree) is loaded. However, this does not mean that the content, such as the actual image content, is loaded. The browser's onload
event, on the other hands, fires after all the content including the images are loaded.
Pop quiz
Q1. Which is the best place to put JavaScript code?
- Before the
<head>
tag - Inside the
<head></head>
elements - Right after the
<body>
tag - Right before the
</body>
tag
Downloading the image assets
We need some graphic files in this step. You can download the graphic files in the code bundle or from http://mak.la/book-assets/.
In the assets bundle, you will find image files for Chapter 2
. After downloading them, put the files in the images
folder. There should be four files, as shown in the following screenshot:
