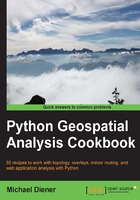
Creating a projection definition for a Shapefile if it does not exist
You recently downloaded a Shapefile from an Internet resource and saw that the .prj
file was not included. You do know, however, that the data is stored in the EPSG:4326 coordinate system as stated on the website from where you downloaded the data. Now the following code will create a new .prj
file.
Getting ready
Start up your Python virtual environment with the workon pygeo_analysis_cookbook
command:
How to do it...
In the following steps, we will take you through creating a new .prj
file to accompany our Shapefile. The .prj
extension is necessary for many spatial operations performed by a desktop GIS, web service, or script:
- Create a new Python file named
ch02_04_write_prj_file.py
in your/ch02/code/working/
directory and add the following code:#!/usr/bin/env python # -*- coding: utf-8 -*- import urllib import os def get_epsg_code(epsg): """ Get the ESRI formatted .prj definition usage get_epsg_code(4326) We use the http://spatialreference.org/ref/epsg/4326/esriwkt/ """ f=urllib.urlopen("http://spatialreference.org/ref/epsg/{0}/esriwkt/".format(epsg)) return (f.read()) # Shapefile filename must equal the new .prj filename shp_filename = "../geodata/UTM_Zone_Boundaries" # Here we write out a new .prj file with the same name # as our Shapefile named "schools" in this example with open("../geodata/{0}.prj".format(shp_filename), "w") as prj: epsg_code = get_epsg_code(4326) prj.write(epsg_code) print "done writing projection definition to EPSG: " + epsg_code
- Now, run the
ch02_04_write_prj_file.py
script:$ python ch02_04_write_prj_file.py
- You should see the following screen output:
done writing projection definition UTM_Zone_Boundaries.prj to EPSG:4326
- Inside your folder, you should see a new
.prj
file created with the same name as the Shapefile.
How it works...
We first wrote a function to fetch our projection definition text using the http://spatialreference.org/ API by passing the EPSG code value. The function returns a textual description of the EPSG code information using the esriwkt
formatting style, indicating ESRI Well-Known Text, which is the format that the ESRI software uses to store the .prj
file information.
Then we need to input the Shapefile name because the filename of .prj
must be equal to the Shapefile name.
In the last step, we'll create the .prj
file using shp_filename
that is specified, and call the function that we wrote to get the text definition of the coordinate reference system.