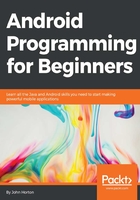
The Android Studio guided tour
Android Studio remembers what you were doing just before you shut it down. So it is possible that when you start it up next, it will initially look a little different from how I show and describe it here. Start reading this guided tour, and it shouldn't take more than a couple of clicks to follow along and inspect all the different areas.
I rarely suggest that it is worth memorizing information, as repeated use and practice of concepts is a much better method to make important ideas take hold. However, on this occasion, it is worth remembering the names of the key Android Studio windows; this is so that you can easily follow along with where the action is when we are doing future tutorials. Of course, we have seen a few of these already (such as the editor and the Properties window), and if you can't remember them all, you can easily refer to this section later.
Tip
Tip of the day
Each time you start Android Studio, you will be presented with a "Tip of the day" message box. It is well worth reading these, as although some of the tips might not make sense at the moment, many of them contain really, valuable information that can either save time or enlighten us in some way.
Parts of the UI
In this next figure, you can see Android Studio open with the Hello Android
app from the previous chapter. Although we are done with this project, it can serve us one more time here.

Let's take a quick look at the different areas that are labeled in the preceding screenshot, and then we can focus on the design views that Android Studio offers us:
- A: This is the menu bar. As with most applications, we can get to almost any option from here.
- B: This is the tool bar, from which you can access one of the many quick-launch icons. From here, some of the most commonly used options can be accessed with a single click.
- C: This is the navigation bar. It shows us the location of the file that is currently open in the editor window within our project and allows us to quickly navigate to a file or folder.
- D: These are the editor tabs. We can click on a tab to see its contents in the editor window. Note that there are more files in our project than are shown in the editor tabs. We can add a file to the editor tabs by double-clicking on them in the project explorer. Refer to H to see the files and folders.
- E: This is the editor and this is where we will spend the majority of our time. Note that although the previous screenshot shows a plain old code file in the editor, when we select a layout file to open, the editor window transforms itself into a mini design studio. And, it also performs some other context-sensitive transformations for other file types, as we will see throughout the rest of the book.
The console
The F window in the preceding screenshot is labeled Android. This is where we have been viewing the logcat output. Note, however, that on the top of this window, there are the following tabs:
- logcat: This is the place where errors, the Log output, and other useful information will appear.
- ADB logs: This is like a virtual console where we can get things done from the command line. There will be no need to do so in this book.
- Memory: Click on this tab when your app is running to see a pretty graph of your app's memory usage. We can use this tab to look for unexpected spikes in memory usage and try and make our apps more memory efficient.
- CPU: Click on this tab when your app is running to see a pretty graph of your app's CPU usage. We can use this tab to look for unexpected spikes in CPU usage and try and make our apps more efficient.
Including the four tabs we just discussed here, there are another four tabs below the window. If you click on one of the following tabs, the Android window changes the window name or divides the current window in two. Here is what you can do with the four tabs that are below the Android window:
- TODO: This shows the TODO comments spread throughout the project's code. This is really useful; try this out: type
// TODO note to self
anywhere in your code. Perhaps like this:// TODO: The flashing pig bug might be caused by the code below!
And no matter how many code files you have, you will see your note and be able to jump to the precise line in the code directly from your TODO window. Think of it as a code comment on steroids. Why not try it now?
- Android: This takes us back to the Android window and the four tabs we discussed previously.
- Terminal: You can use this to navigate your OS and do anything you can do from a console (DOS) window.
- Messages: As the name suggests, you will get system and error messages in this window.
More console features
At the part labeled G, in the corner of the preceding screenshot, we have two more tabs: Event log and Gradle console. Immediately below the two tabs is the status bar. When Android Studio is busy doing something for us, it will let us know here. So if things seem a bit unresponsive or you are not sure whether you actually clicked on the play icon, then check here first before clicking on it again.
Now let's look at the two tabs in detail:
- Event Log: Every time Android Studio completes a significant event, it is logged here. So if you can't remember the steps you have taken so far or whether a particular process has been completed successfully, you can check here.
- Gradle console: Gradle is a build system. Without us even realizing, Android Studio has been using Gradle to automate the process of turning the files in our project into an app that can be run on a device. This console allows you to give the Gradle tool a few commands and see it's response. We won't need to do this, however.
Tip
If you want to learn more about Gradle and go beyond the knowledge you need to complete this book, you can do so with this exploratory tutorial: http://www.vogella.com/tutorials/AndroidBuild/article.html
The project explorer
The part labeled as H is the project explorer. This is essentially a file browser. We will explore the file and folder structure of our project in the next section, but just know that you can navigate to a file in this window and double-click on it to open it and add a tab for it to the editor window.
Note that there are a number of tabs down the left-hand side of Android Studio that cause the project explorer window to be replaced. Here is a quick run down of what the main ones do, starting from the top:
- Project: Click on this tab to switch back to the project explorer we just discussed.
- Structure: Here, we can see the hierarchy of our project broken down into classes, methods, and other components. Then, we can simply click on a part of the hierarchy to be taken to the selected part of the code in the editor window.
- Build variants: Android Studio enables us to test multiple different versions—builds—of our app. This isn't something that we need to discuss in this book.
- Favorites: Through this, you can see all the places that you have visited the most, bookmark files, or the points in your code that you have been debugging.
Transforming the editor into a design studio
I declined to label all the possible tab options around the edge of the UI because there are so many of them and as we saw, sometimes exactly which tabs you can see depends upon the context and the view that is currently occupying the given window. We gave most of the tabs a mention in this quick tour when we talked about the window that is nearest to them.
One set of tabs that does require further coverage are the tabs that contain the open files (D). The reason for this is that the editor window (E) completely transforms itself depending upon what file type is open in it. We have already seen the editor window transform itself when we switch between our Java code and our layout file.
As a reminder, click on the my_layout.xml tab (D). Next, make sure that we have the main design view by clicking on the Design tab at the bottom-left corner of the editor window. Here is another labeled screenshot to make sure that we are referring to the right parts of the editor window when in the design view:

We will spend most of our time, in the next few chapters, in the design view of the editor window. If the previous screenshot looks slightly different than your layout, then you can take the following steps to make your layout more design friendly:
- Make the editor window larger by making sure that the Android window is closed. Click on the Android tab if the window is open, and this will make it disappear.
- Make the project explorer window smaller by dragging the area between it and the editor to the left.
- Make the Palette area (E2) larger by dragging its right-hand side edge to the right.
- Tweak all the window sizes to suit your preferences.
You will note that all the windows we discussed right from A to H are still present/available, and it is just the editor window that has been radically transformed. Let's have a look at what the editor window has to offer by following the labels on the previous screenshot.
E1 – the Preview toolbar
This small, but useful, area of Android Studio is certainly worth talking about. I won't cover every button, but here are some of the highlights. If you want to see what your layout will look like on a particular phone or tablet, click on the virtual device drop-down list and select the device of your choice, as shown in the following screenshot:

You can also click on the button pictured as follows to rotate the preview to landscape. Click on the button shown here to try this out:

As you might expect, this will make our layouts look significantly different. We will start to see how we can handle this later in the chapter and throughout the book.
We can zoom in and out of our layout design with the buttons shown here:

And the button shown next is the refresh button, which is just like the refresh button in a web browser. It forces the preview of our layout to be updated in case it doesn't happen automatically.

We haven't covered every button or control, but we have seen enough to move on.
E2 – exploring the palette
Next, we have the Palette window. The palette contains dozens of different design elements that we can drag onto our layouts. These are divided into categories and are all covered in the following sections. The key categories for this chapter are widgets and layouts, so we will cover them more thoroughly than the rest. Take a look at this close-up shot of the palette:

Let's go through some of the key sections of the palette and later, we will begin to actually use them:
- Layouts: Layouts are, as their name suggests, used to lay out all the other elements within them. What is key, however, and will become apparent as we progress, is how different layouts are more suited to different situations. In addition, we can use the same widgets on different types of layouts, and the XML code that will be generated for us will vary quite a lot. This will not be a problem for us because we will look at lots of examples, and as we will see later, we do not need to remember the different syntax for each layout type; we just need to be aware of the different situations suited to each layout type. Also, we will regularly use multiple layouts as part of the same design. That is, there will be layouts within layouts. Just think about putting storage containers of different shapes and sizes within bigger containers. It's the same concept. You will learn through practice about which containers are best for which contents in different situations.
- Widgets: Are the most commonly used elements on the palette. Typically, there will be multiple widgets contained within a layout. Widgets are the part of our layout that the user will most often see and interact with. We have already seen the Button and PlainTextView widgets.
- Text Fields: These are like PlainTextView from the Widgets category, but are very specific to the type of text that they are most suited to and are most often used when the user actually interacts with or changes the values that they hold. Take a look at the names of the elements in the Text Fields category and you will see that they all have names that allude to their likely use.
- Containers: Containers are like layouts with a specific purpose. For example, the Radio Group container will hold multiple Radio Button elements from the widget category. The ScrollView container will hold a whole bunch of other elements and enable the user to scroll through them. VideoView is a fast and easy way to allow the user to have a fully functional video player with little to no coding. We will see some of these containers in action later in the book.
- Date & Time: Ever wondered why the pop-up date or calendar selector looks so similar on so many apps? That's because they are using the Date and Time elements.
- Expert: These elements are fairly diverse from each other. We will see some of the elements from the expert category throughout the book. For example, in Chapter 28, Threads, Touches, Drawing, and a Simple Game, we will see SurfaceView in action when we take a whirlwind tour of how to make animated 2D games for Android.
- Custom: We can think of these as the building blocks of specialized layout elements. Fragment is probably the most powerful and versatile of all the layout elements, and we will spend the majority of latter part of this book taking advantage of it.
After that fast overview of the palette, let's move on to E3.
E3 – the layout preview
This is the layout preview where we will preview our masterpieces. Take a closer look at E1 to see how we can switch between landscape and portrait, refresh or zoom the preview, and change the virtual device. And here is a close-up view of E3, which shows the layout preview just after a new project is started:

As we will see when we take a look at the area labeled E6, we can view our layouts/designs visually or as code.
E4 – the Component Tree
This is the Component Tree. It can be a UI designer's life saver. As our layouts get more complicated with layout elements nested inside other layout elements and widgets all over the place, the XML code as a whole can become awkward to navigate. The Component Tree allows us to see the structure as well as the individual elements of our design. We can expand and collapse parts of it; jump to specific sections of the XML code; as well as drag, drop, and rearrange parts of our design with it.
E5 – the Properties window
This is the Properties window. We have already been here when we were editing the properties of our widgets back in Chapter 2, Java – First Contact. We have seen that we can edit properties in both the XML code and the Properties window. There is no right or wrong way to do this and, most likely, you will end up using both. Where the Properties window can be really helpful, when we are just starting to program for Android, is in two areas. One obvious one less so. The obvious advantage is that we don't have to mess around with all that nasty-looking XML code. The other advantage is that, even when we get comfortable with the XML code, it is going to be a long time before we remember the names of all the properties. The Properties window allows us to browse through all the available properties for the selected element. When we add a property, it will, of course, add the full line of XML, negating the need for us to remember what the property was called and the convoluted syntax needed to add it.
E6 – text and design view tabs
Here, we can see the tabs that are used to switch between the Text (XML) view and the Design view. These tabs are only visible when we are viewing a file that has these views available. For example, when we are viewing our Java code, these tabs will not be present.
Let's talk some more about the project folder and file structure now.
The project folder and file structure
Let's take a closer look at the files and folders that are part of our project. Open up the Hello Android
app from earlier to follow along. Android is a fussy thing, and it likes all of the different resources (layout files, code, images, sounds, and so on) to go in the correct folders or it will complain and not compile or run correctly.
Android Studio, with the help of Gradle, keeps track of the resources in our project and can advise us when we are trying to use something that doesn't exist or if we've misspelled the name of an image or a sound file, for example. Almost instantly after adding a resource to the project, Android Studio will 'know' about it and make it available to us.
If you expand all of the folders in the project explorer, even from our simple project from Chapter 1, The First App, you will see a vast array of files and folders.
If the vastness and depth of some of the files and folders is a bit intimidating, then you will be pleased to learn that we will never need to look at or edit most of them. Most of them are managed by Android Studio. We will focus on the key folders and file types that we will be using as Android developers.
Expand the following folders (if they aren't already) by clicking on the little triangle to the left-hand side of each of them, once each on: app
, src
, main
, java
, res
, main
, and layout
folder. You will see something close to this next figure. This figure is a close-up view of the folders we are most interested in. As usual, I have labeled the most useful parts, so we can refer to them and describe them.

We can find almost every file and folder we will need in the app/src/main
folder as indicated by (1).
Note that in the java
folder (2), inside a subfolder with the same name as the package for this project (com.gamecodeschool.helloandroid
), we can see our MyActivity.java
(3) code file. Android Studio hides the file extension for .java
files, but being inside the java
folder should make it plain what we are dealing with. If we create two or more different packages in a project, then the subfolders with the names of the packages will appear in the java
folder and, of course, will contain their .java
files as we might expect.
The other major thing we will be constantly adding to and editing in our Android projects is resources. These are all contained within the res folder (4). As we saw in Chapter 1, The First App, the variety of resources our projects can use is wide. Let's see where some of them go.
The drawable
folder (5) is where all the graphical assets go. If we design a fancy button, background, or icon, then this is the place for it. In addition to this, we will create subfolders within the res
folder that contain scaled versions of our graphical resources. These subfolders are used by devices with varying screen densities and sizes and have names such as drawable-hdpi
, drawable-mdpi
, drawable-xhdpi
, and drawable-xxhdpi
.
These folders will contain equivalent graphical resources for devices with high (h), medium (m), extra high (xh), and extra extra high (xxh) dpi screens. This is optional because Android will scale any graphics where a version specific to the current screen type is not provided; however, this often results in a stretched or low-quality appearance.
Inside the layout
folder, we can find our my_layout.xml
(6) file, which, of course, contains the layout from our project. We can have more than one layout in a project and when we create them, they will appear in this folder. As an example, we might have a project with home_layout.xml
, settings_layout.xml
, and main_layout.xml
. In addition to this, just like with the drawable
folders, we can have subfolders within res
for the layouts of devices with different dpi screens. The names are fairly predictable, but for the sake of clarity, they would be layout-hdpi
, layout-mdpi
, and so on.
We can also add folders that handle different orientations such as layout-landscape
and layout-portait
.
Furthermore, we can add folders that handle the screen size as opposed to density, such as layout-large
, layout-xlarge
, and more.
Tip
Folders galore
All this talk of folders might be making your brain ache. The first thing to note is that, as we already mentioned with the drawable
folder, the extra folders are always optional. You can easily build an app with only one set of graphics and layout files. In fact, the techniques we learn in the latter part of this book reduce the need for dozens of different folders in the res
folder. However, being aware of devices of different sizes/dpi's, testing on the related emulators, and catering for them when necessary will make your app truly versatile with a consistent appearance. Now for the bad news; there are even more screen qualifiers than these main qualifiers that we mentioned here. If you want to take a look, you can do so at http://developer.android.com/guide/practices/screens_support.html. We will make some use of these different folders throughout the book and deal with them in a step-by-step manner when we do.
Moving on to number 7 on our folder structure diagram, we have the values
folder. This contains the strings.xml
file. Note that the name of our app is not mentioned anywhere in our Java code. The reason for this is that although you can hardcode text into your Java code or a layout file, it is much better to use string resources in the strings.xml
file. Here is why. String resources are the indexed text (words and phrases) that the user sees in our app (such as Hello world!
) contained in the strings.xml
file.
Let's say you have a settings screen and on at that settings screen layout, you hardcode the words Settings, Volume, and Theme. No problem so far. Now consider that your app might have multiple different screens and each of them has a button that the user can click on to go to the Settings screen. That's OK, just hardcode the text in the buttons as well. Now imagine that the lead designer decides that the Settings screen should be called Options and not Settings, and the app is also going to be released in the German market, which will require all the strings changed to German, of course. Now consider that your app has more than just a settings screen; perhaps, it has pop-up tips on how to use your app. Even something as apparently straightforward as the app's title might not only be different when translated to other languages but might even be different, perhaps for cultural reasons in some countries.
By using string resources, we can manage this problem simply. Take a look at the strings.xml
file in the values
folder by double-clicking on it. Here are the contents for your convenience. Note that I have highlighted one line of code in the following snippet:
<resources>
<string name="app_name">Hello Android</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
</resources>
Now look at this next figure that shows a line of code on TextView
in the my_layout.xml
file from the Hello Android project:

In the previous figure, I was hovering the mouse cursor over the phrase "Hello world!". Note that the code reads text="Hello world!"
, but the pop up shows that actually the phrase is not contained directly in the TextView
tag itself, but is being referenced in the strings.xml
file via the @string/hello_world
identifier.
So, string resources allow us to define the text that appears in our app and then refer to its index in the strings.xml
file. This makes problems such as words and phrases that appear multiple times or translating to new languages more manageable.
Doing things this way does seem longwinded for one simple phrase, but when we consider the previous scenario of the settings screen, then the advantages are plain. Throughout this book, we will hardcode and use string resources depending on which seems most appropriate for the situation. Generally, when implementing a complete project, we will do things properly, and when we are making a simple app just to learn a concept, we will cheat and hardcode our Strings.
Tip
All is not as it seems
If you use your operating system's file browser and browse to the project folder, then you will see that things are quite different than how they appear in the project explorer. There are many more subfolders than the project explorer reveals. The project explorer not only gives you access to all the files and folders you need, it also simplifies their location and un-buries them a little. The real file and folder structure is required by Android, so we mustn't try and change it. So performing all the editing, moving, and adding directly through Android Studio is a good idea.
It is not necessary to memorize everything we have said about files and folders. Each time we need to do something with a folder or file, I will be really specific about what to do. It is just helpful to have an overview of the structure that Android needs to work properly. Just think of Android as a fussy (but loving) flatmate who absolutely must have a place for everything and everything in its place.