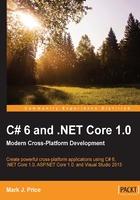
Checking for overflow
Earlier, we saw that when casting between number types it was possible to lose information, for example, when casting from a long
variable to an int
variable. If the value stored in a type is too big, it will overflow.
Add a new Console Application project named Ch03_CheckingForOverflow.
The checked statement
The checked
statement tells .NET to throw an exception when an overflow happens instead of allowing to it happen silently.
We set the initial value of an int
variable to its maximum value minus one. Then, we increment it several times, outputting its value each time. Note that once x
gets above its maximum value, it overflows to its minimum value and continues incrementing from there.
Type the following code in the Main
method and run the program:
int x = int.MaxValue - 1; WriteLine(x); x++; WriteLine(x); x++; WriteLine(x); x++; WriteLine(x);
Press Ctrl + F5 and view the output in the console:
2147483646 2147483647 -2147483648 -2147483647
Now let's get the compiler to warn us about the overflow using the checked
statement:
checked { int x = int.MaxValue - 1; WriteLine(x); x++; WriteLine(x); x++; WriteLine(x); x++; WriteLine(x); }
Press Ctrl+F5 and view the output in the console:
2147483646 2147483647 Unhandled Exception: System.OverflowException: Arithmetic operation resulted in an overflow.
Just like any other exception, we could wrap these statements in a try-catch
block and display a nicer error message for the user:
try { // previous code goes here } catch(OverflowException) { WriteLine("The code overflowed but I caught the exception."); }
Press Ctrl + F5 and view the output in the console:
2147483646 2147483647 The code overflowed but I caught the exception.
The unchecked statement
A related keyword is unchecked
. Type the following statement at the end of the previous statements. The compiler will not compile this statement because it knows it would overflow:
int x = int.MaxValue + 1;
Press F6 to build and notice the error, as shown in the following screenshot:

Note that this is a compile-time check. To disable compile-time checks, we can wrap the statement in an unchecked block, as shown in the following code:
unchecked { int x = int.MaxValue + 1; WriteLine(x); x--; WriteLine(x); x--; }
Press Ctrl + F5 and view the output in the console:
2147483646 2147483647 The code overflowed but I caught the exception. -2147483648 2147483647 2147483646
Of course it would be pretty rare that you would want to explicitly switch off a check like this because it allows an overflow to occur. But, perhaps, you can think of a scenario where you might want that behavior.