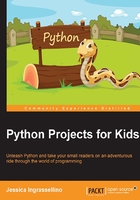
New functions – subtraction, multiplication, and pision
Now that we have created an addition()
function that accepts data from the user and converts it into numbers, we are ready to create functions for subtraction, multiplication, and pision.
If you are coming back to this after a break, perform the following steps:
- Open your Python shell so that you can test your code as you write.
- Next, open your text editor (jEdit in Mac/Linux and Notepad ++ in Windows).
- Have both windows open on your desktop as you program.
- When you successfully write and test a line or a few lines of code in the Python shell, copy the lines into your text editor and then Save Your Work to the
first_calc.py
file that you created earlier in this chapter.
Tip
Save your work early and as often as you can! Avoid being upset by accidentally erasing your code!
Subtraction
For the next part of our calculator, we will make our subtraction function. We will follow the same prompts as we used for the addition function to create a second function that performs subtraction. In your Python shell, try these steps to create the subtraction function:
- Type
def
to start your function. - Name your function.
- Add proper syntax, which is parenthesis
()
and:
. - Tab the remaining lines in four spaces each.
- Request the first number from the user.
- Request the second number from the user.
- Print the output using the minus (
-
) symbol for subtraction.
Once you have tried creating this function in the Python shell, try calling the function using this line of code:
subtraction()
If the function call works, then you can type your code into your code file exactly as it appears in your Python shell. If your subtraction()
function does not run, make sure you did not make any errors when typing your code in the shell. Double-check your code and rerun it until it is smooth. If you are stuck, you can copy the lines of the following code into your Python shell; they will perform subtraction on two integers:
def subtraction(): first = int(raw_input('What is your first number?')) second = int(raw_input('What is your second number?')) print(first - second) subtraction()
Once you have tested your code in the shell, you can then type it into your text editor. Remember to save your work in your first_calc.py
file. Your first_calc.py
file should now look something like this:

Multiplication
By now, you might have observed a pattern in our functions. The multiplication function will follow the same format and logic rules as the addition and subtraction functions. You can continue to ask the user to enter each number, and then the computer will perform a proper calculation.
The following code is for the multiplication function. You can copy it directly, but it is a better idea to try to create the multiplication function on your own. If you try to create your function, you will know how well you have learned the way to create a function. When you are ready, you will see this code for the multiplication function:
def multiplication(): first = int(raw_input('What is your first number?')) second = int(raw_input('What is your second number?')) print(first * second)
Once you have tested your code in your Python shell, remember to type the function in your text editor and save your work in your first_calc.py
file:

Division
Division is the final basic operation that we will program for our first calculator program. As with multiplication, you have already done most of the work for the pision part of the calculator. See if you can recall how to create a pision function from scratch. Once you have tested your code, compare it to the following code and see if it matches up:
def pision(): first = int(raw_input('What is your first number?')) second = int(raw_input('What is your second number?')) print(first / second)
Once you have tested your code, remember to save your work in your first_calc.py
file:
