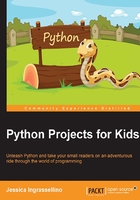
Functions
Once we have variables, we can use them to do some pretty interesting things. The most interesting thing is to build functions. Python functions are blocks of code that we can build to do a specific job. We build these functions once, and then we can reuse them in our code just by typing the name. This is really helpful. For example, if I need to write a program that adds two numbers (a calculator, for example), I do not want to have to write three or four lines of code every time I want to add two numbers. Instead, I want to write one function that can add two numbers together, and then use that single line whenever I need to add numbers.
Before we begin building functions of our own, we need to also know that Python has a lot of amazing functions that are built in. Some of Python's functions are things we will use all the time. Others we won't talk about in this book, but as you become a more skilled programmer, you will learn more about Python's built-in functions.
Built-in functions
Here's something about some built-in functions and what they do:
int()
: This converts a string or a float into an integerfloat()
: This converts a string or an integer into a floatraw_input()
: This gets information from a user and stores it in the computer to use laterstr()
: This converts an integer, float, or other information into a stringhelp()
: This provides access to Python's help
We will be using these functions to help us build our first project in the next chapter.
Note
If you are curious about what other functions are built in or if you want to know more, you can go to the Python documents at https://docs.python.org/2.7/library/functions.html?highlight=built%20functions#.
Initially, the documents can seem overwhelming because they are very detailed. The detail can make the documents difficult to understand at times, but the documents are very helpful and are used by many programmers.
Parts of a function
There are basic parts to think about when you want to build your own function. First, here's the basic function to add two numbers:
def addition(): first_number = 30 second_number = 60 print(first_number + second_number)
The first line of this code is new, so we need to understand what it means:
- The first thing to notice is the word
def
. In Python, this is short for define, and it is used to define a new function. - The next thing to notice is the name of the function. The name of the function has the same guidelines as the names of variables. A function needs to use lowercase letters, and when it has many words, there need to be underscores between each word.
- After the name of the
addition()
function, you will notice the parentheses()
. These are empty in this function, but sometimes they are not empty. Even if they are, the parentheses must ALWAYS be a part of the function that you create. - Finally, the first line of the function ends with a colon. The colon (
:
), ends the first line of the function.
A function can be short, such as this addition()
function, which is only four lines in total, or it can be really long. Every line after the first line in a Python function needs to be indented using spaces. When we work on building our own functions in the next section of this chapter, you will learn how to make indents in your Python shell. We will also discuss proper spacing in the text editor.
There are a lot of new details to remember in order to write a function. What happens if you forget a detail? If you forget to indent a line, Python will let you know and output an error. Your function will not run, and your code will not work. Python's use of indentation is known as whitespace, and there are rules about whitespace use in Python.
By now, you are very familiar with doing additions in Python, so we will keep working with the addition code. There are special considerations in order to write functions in the Python shell. Because a function is a block of code, we want to follow these guidelines when we are trying to perform functions in the shell:
- After you type the first line and press Enter, make sure you press Tab before you type each line
- When you have completed typing all the lines, hit Enter twice so that the Python shell knows that you are done creating the function
In your Python shell, type the addition()
function exactly as it appears here:
def addition(): first_number = 30 second_number = 60 print(first_number + second_number)
Notice how the function looks in the Python shell:

Now that you have typed your function, you need to learn how to use the function. To use the function in the Python shell, type the name of the function and the parentheses:
addition()
Typing the function is also known as calling the function. When you call the addition()
function in the Python shell and then press Enter, you will get an output as the answer to the problem. Notice how this is displayed here:

Compare your result to the results shown in the preceding screenshots. Once you know that your function runs the way you want it to, you can show it to your parents and friends by asking them to look at your code. It is a good idea to test your function by retyping it with different numbers.