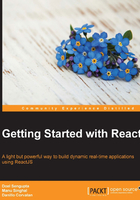
Why JSX?
In general, HTML and JavaScript are segregated in frameworks by defining UI or a view to represent their mutable data, which normally is a template language and/or a display logic interpreter. The following is some jQuery code:
<html> <head> <title>Just an example</title> </head> <body> <div id="my-awesome-app"> <!-- Here go my rendered template --> </div> <script id="my-list" type="text/html"> <ul> {{each items}} <li> ${name} </li> {{/each}} </ul> </script> </body> </html>
The script
element represents a template component that will be rendered in the my-awesome-app div
element. The code here is a JavaScript file that pushes data to that template and asks jQuery to do the job and render the UI:
$("#my-list").tmpl(serverData).appendTo("#my-my-awesome-app");
Whenever you want to put some display logic on that code, you will need to rely on both JavaScript and HTML files. In other words, a single component is a mix of files—normally, a JavaScript file that controls the view, a template/markup file representing the view, and a model/service that fetches data from the server and sends it to the view. Typically in an MVC application, the logic of M(model), V(view), and C(controller) are separated in order to provide the separation of concern and better readability and maintenance of the code.
Let's say that we now have to change this view and need to hide the list when the user is not logged in. Considering that the model/service code is already bringing this information, we'll have to change both the code that controls the view and the markup one in order to apply those changes. Harder the change is, more painful it is to apply those changes. Our code ends up in big JavaScript and HTML files, mixed up with display logic, template expressions, and business code.
Although you are an experienced frontend developer, apply some separation of concerns, and split your UI into smaller views, you end up with hundreds of files just to represent a single piece of UI: view controller, HTML template, style sheet, and your model. It makes a small application look complex with that amount of files, and you'll certainly get messy wondering which file is part of a specific view or component.
The thing we want to show here is that we've been mixing markup and logic code since the beginning, but other than that, we've also been splitting them into other files, making it more difficult to find and to modify them.
ReactJS with JSX drives you in the other way. There is a really interesting paragraph in the ReactJS official page that honestly reasons this powerful library and its paradigm:
"We strongly believe that components are the right way to separate concerns rather than "templates" and "display logic." We think that markup and the code that generates it are intimately tied together. Additionally, display logic is often very complex and using template languages to express it becomes cumbersome. (http://facebook.github.io/react/docs/displaying-data.html#jsx-syntax)
We like to think of ReactJS components as a single source of truth. All other locations that use your component will be just references. Every change you apply to the original one will be propagated to all other places referencing it. Customization is easily done through properties and child componentization. JSX is like a middleware that converts your markup code to objects where ReactJS can handle them.
JSX speeds up the frontend development in ReactJS. Instead of creating literal objects to represent your UI, you create XML-like elements very similar to HTML, and you can even reference other components that you've created. Also, it's very straightforward to reuse third-party components or even publish your own. In a corporate environment, you could have a commonly used components repository that other projects can import from.
Tools for transforming JSX
The JSX Transformer file and other tools, as already mentioned, are responsible for transforming your JSX syntax into plain JavaScript. The ReactJS team and the community provide some tools for that. Such tools can deal with any kind of file since they have JavaScript code and JSX syntax. In older versions of React, a comment was required on the first line of .js
files such as /** @jsx React.DOM */
. Thankfully, this was removed after version 0.12.
JSX Transformer has been deprecated now. Instead, we can use https://babeljs.io/repl/ to compile the JSX syntax into JavaScript. To include JSX in your script tag, either use <script type="text/jsx">
or while transforming, use babel
<script type="text/babel">.
Earlier there was an online tool at http://facebook.github.io/react/jsx-compiler.html. However, the React developer team discontinued it, and JSX Transformer has been deprecated.
Since such JSX transformation would take a substantial computation at the client side, we should not be doing these transformations in production environments. Instead, we should use:
npm install -g bab el-cli

We can also use the node npm
package that the ReactJS team built to transform your JSX files. First, you need to install the react-tools
NPM package with:
npm install react-tools –g
This will install react-tools
globally. All you need now is to run the following command from your project folder:
jsx --watch src/ build/
This command transforms every script in the src
folder and puts it in the build
folder. The watch
parameter makes this tool run the same command every time a file changes in the src
folder. This is a very useful tool because you're using node to bundle your frontend code.
If you're familiar with task runner tools such as Grunt or Gulp, they also have JSX transformer packages that can be installed with npm
as well. In this case, they provide more options that can fit better in our deployment/building process, mainly if you already use one of them. It's not the purpose of this book to dive into Grunt or Gulp. In order to configure and install them, you can follow their guidelines in the following links: Details of these are covered in Chapter 9, Preparing Your Code for Deployment.
- Grunt – https://www.gruntjs.com
- Gulp – https://www.gulpjs.com
Both sites have a /plugins page where you can search for available plugins. The following are the links of these download tools:
- Grunt React task—https://www.npmjs.com/package/grunt-react
- Gulp React task—https://www.npmjs.com/package/gulp-react/
They work much the same as do the React tools. We are going to use the transformer
script file that is placed in head
the element of our HTML page for the next examples, as this is easier to do. In Chapter 9, Preparing Your Code for Deployment, we are going to use webpack
and gulp
as the npm
packages to transform our JSX code and prepare it for deployment.