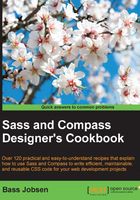
Using nested selectors
In contrast to vanilla CSS code, Sass allows you to nest selectors. Nesting of selectors may help create better organized and more readable CSS code.
Getting ready
You can use the Ruby Sass compiler to compile the Sass code in this recipe into CSS code. Read the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, to find out how to install Ruby Sass. Use a text editor, as described in the Writing your code in a text editor recipe of Chapter 1, Getting Started with Sass, to edit your SCSS code.
How to do it...
The following steps will show you how to use nested selectors in Sass:
- Create a Sass template called
main.scss
that will contain the following SCSS code:// scss-lint:disable ColorKeyword $text-color: black; $link-color: red; p { color: $text-color; a { color: $link-color; } }
- Compile the
main.scss
file from the previous step into CSS code by running the following command in your console:sass main.scss
- You will find that the CSS code outputted to your console will look as follows:
p { color: black; } p a { color: red; }
How it works...
Because nested selectors in Sass can follow the structure of your HTML code, your CSS code will become more easy to read and maintain. Another advantage of nesting your selectors is when you change the name of a selector/element; you only need to change it in one place (the outer selector) instead of in numerous places, like you would when writing CSS. The SCSS for a certain HTML element or structure becomes portable and can be reused in more than one project.
When inspecting the compiled CSS code from the last step of this recipe, you will find that the nested SCSS code compiles into unnested and valid CSS code. The a
selector, which has been nested inside the p
selector, compiles as expected into a p a
selector.
There's more...
We call the compiled p a
selector in the compiled CSS code of this recipe a descendant selector. The preceding descendant selector selects all the a
selectors that are nested anywhere in a p
selector of your HTML markup structure.
Other types of selectors supported by CSS are the child combinator, the adjacent sibling combinator, and the general sibling combinator. The following SCSS code shows how you can easily build these selectors with Sass using nesting too:
// scss-lint:disable ColorKeyword $light-color: green; $default-color: red; $dark-color: darkred; nav { ul { li { // descendant selector color: $light-color; } > li { // child combinator font-size: 1.2em; } } ~ p { // general sibling combinator color: $default-color; } + p { // adjacent sibling combinator color: $dark-color; } }
The preceding code compiles into CSS code as follows:
nav ul li { color: green; } nav ul > li { font-size: 1.2em; } nav ~ p { color: red; } nav + p { color: darkred; }
Also, pseudo classes can be nested in your SCSS. You can read more about nesting pseudo classes in the Referencing parent selectors with the & sign recipe of this chapter.
Using nesting to group-related selectors together makes your Sass code more readable. But when you nest too deep, your code becomes less portable. In the Avoiding nested selectors too deeply for more modular CSS recipe of this chapter, you can read more about the best practice for nesting.
See also
Read also the Child and Sibling Selectors by Chris Coyer at https://css-tricks.com/child-and-sibling-selectors/ to learn more about the selectors discussed in this recipe.