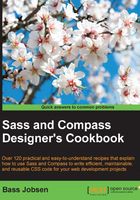
Duplicating mixins and name collisions
When you define mixins with the same name, you may possibly get conflicting code. This recipe explains how to prevent name collisions in Sass.
Getting ready
Read the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, to install Ruby Sass on your system. This recipe uses Ruby Sass to compile your CSS code. The SCSS code can be edited in your favorite text editor.
How to do it...
The following steps will demonstrate you how duplicate mixin names may influence the compiled CSS code:
- Create a Sass template called
mixins.scss
. This file should contain the SCSS code like that shown here:// scss-lint:disable PropertySpelling @mixin mixin { property: first; } s1 { @include mixin; } @mixin mixin { property: second; } s2 { @include mixin; }
- Compile the SCSS code from the first step by running the following command in your console:
sass mixins.scss
- The command outputs the following CSS code in your console:
s1 { property: first; } s2 { property: second; }
How it works...
In this recipe, two mixins with the same name are defined. As you can see, the second mixin overrides the first one, but only for calls after the second's declaration.
Overriding of mixins can be complicated when using more than one mixin library.
Collision of class names in the compiled CSS code can be prevented by wrapping the @import
directive inside a class selector, as shown next. You can read more about the @import
directive in the Importing and organizing your files recipe of Chapter 5, Building Layouts with Sass:
.bootstrap { @import 'bootstrap'; }
The preceding SCSS code wraps all the classes compiled by @import 'bootstrap';
in the .bootstrap
class, so you can use the compiled classes and selectors together with the .bootstrap
class in your HTML. Bootstrap's mixins are not reusable and selectors cannot be extended with the @extend
directive. Read more about Bootstrap in Chapter 12, Bootstrap and Sass.
The Scut mixin library prevents the earlier mentioned problem with conflicting names by adding a prefix to every class and mixin. Sass functions, as described in the Creating pure Sass functions recipe of this chapter, should also be prefixed to prevent name collisions.
There's more...
Sass also does not support the overloading of mixins. You can use default values as parameters to make a mixin callable with a different number of arguments. Read the Writing mixins with arguments recipe to learn more about parametric mixins. The following example shows you how to create a mixin with default values:
@mixin transparent-backgrounds($color, $opacity: 1) { background-color: $color; opacity: $opacity; }
Now, you can include the preceding mixin with both the @include transparent-backgrounds(blue)
and the @include transparent-backgrounds(blue, 0.5)
declarations. The opacity level describes the transparency level of an element. The opacity level ranges from 1
(not transparent) to 0
(completely transparent). The default value for the opacity
property is 1
. So, when using 1
as the default value for the mixin too, the effect will be the same as not setting the opacity.
Most CSS properties have a default value and the default value for many of them is initial
(the specified value that is designated as the property's initial value; this value does not have to be the browser default) or inherit
(inherit the value from the parent). Also, the initial
and inherit
values can be used as the default value for a mixin.
The recipe also makes clear that the compiler tests the SCSS/CSS syntax, but does not complain about the nonexisting or wrongly spelled properties. The property
property used in this recipe is not defined in the CSS(3) specifications. The SCSS linter tool described in the Writing your code in a text editor recipe of Chapter 1, Getting Started with Sass, also checks for naming errors and gives a warning for the unknown properties. Neither the compiler nor the linter tool throws an error or warning when assigning an invalid value to a property. You will need a CSS validator to check for nonsyntax-related CSS errors.
See also
- Scut is a collection of Sass utilities to ease and improve our implementations of common style-code patterns. You can find the Scut library at http://davidtheclark.github.io/scut/.
- PrettyCSS is a CSS3-compliant parser, lint checker, and pretty printer. With this tool, you can check for CSS' format violations and then beautify the code to standardize it. You can find PrettyCSS at https://www.npmjs.com/package/PrettyCSS.