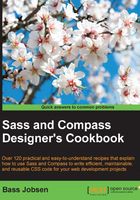
Commenting your code in the SCSS syntax
Commenting your code will help you and others to better understand the code. Comments can also be used by other tools. If you or someone else has to change your code, maybe after a long period since the code has been written, comments should make clear what a block of code does and why it has been added in the first place.
Getting ready
For this recipe, you will only have to install Ruby Sass. The Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, explains how you can install Ruby Sass.
How to do it...
Perform the following step to find out the differences between the three types of comments supported by Sass:
- Create a Sass template called
comments.scss
. In this file, write down the SCSS code as follows:// start comments $author: 'Bass Jobsen'; // scss-lint:disable Comment /*! * Copyright 2015 #{$author} */ // scss-lint:disable PropertySpelling //compilles into invalid CSS .code { author: $author; } // scss-lint:disable Comment /* * this comment does compile into the CSS code unless a compressed output style is used. */
- Now run the following command in your console:
sass comments.scss
- The preceding commands output the CSS code, as follows:
/*! * Copyright 2015 Bass Jobsen */ .code { author: "Bass Jobsen"; } /* * this comment does compile into the CSS code unless a compressed output style is used. */
- Then, run the preceding command with the
--style compressed
option in your console, as follows:sass --style compressed comments.scss
- Finally, you will find that the compiled CSS code looks as follows:
/*! * Copyright 2015 Bass Jobsen */.code{author:"Bass Jobsen"}
How it works...
Sass supports three types of comments: single line, multiline, and special. In this recipe, all three types of allowed comments are used and demonstrated. Multiline comments written between /* */
are part of the official CSS syntax. Multiline comments are compiled in the CSS code unless the output's style is set to compressed
. You can read more about these output styles in the Choosing your output style recipe of Chapter 1, Getting Started with Sass.
Single line comments starting with //
can be used for developers to help them understand the code. Single line comments are not compiled into the CSS code.
Special comments are written between /*! */
. They will always be rendered into a CSS output even in the compressed output modes. You can, for instance, use special comments to add a copyright notice at the beginning of your style sheet. The special comment syntax is also supported by most postprocess minifiers, such as clean-css
. You can read more about these minifiers in the Automatically prefixing your code with Grunt recipe of Chapter 16, Setting up a Build Chain with Grunt.
There's more...
Comments are not always only intended for developers; you can also use comments to instruct the compiler or other computer programs. Some examples are mentioned in the following text.
In the Using CSS source maps to debug your code recipe of this chapter, you have already seen that the source map reference used by the browser is added to the compiled CSS code by using a comment. A source map comment will look like the following:
/*# sourceMappingURL=main.css.map */
In the Writing your code in a text editor recipe of Chapter 1, Getting Started with Sass, you can read about the scss-lint
tool. Special comments help the scss-lint
tool to ignore some checks for some parts of your code. In this recipe, single line comments, such as the // scss-lint:disable PropertySpelling
comment, are used by the scss-lint
tool.
The last example can be found in the Building styleguides with tdcss.js recipe of this chapter. The tdcss.js tool uses comments in your code to automatically build a style guide.
In the Interpolation of variables recipe of Chapter 3, Variables, Mixins, and Functions, you can read about variable interpolation. You can apply variable interpolation to your comments too, which can be used in the recipe to set the author's name.
The following code is from the recipe:
$author: 'Bass Jobsen'; // scss-lint:disable Comment /*! * Copyright 2015 #{$author} */
This Sass code will compile into the CSS code, as follows:
/*! * Copyright 2015 Bass Jobsen */
As you can see, the #{$author}
code has been replaced by the author's name assigned to the $author
variable. Get a grip on variables by reading Chapter 3, Variables, Mixins, and functions, of this book.
See also
Some thoughts on whether to comment or not to comment your code by Neil McAllister can be referred to at http://www.javaworld.com/article/2078485/core-java/how-to-get-developers-to-document-their-code.html.