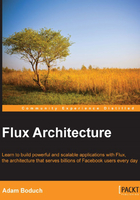
General organization
As a first step in building a skeleton Flux architecture, we'll spend a few minutes getting organized. In this section, we'll establish a basic directory structure, figure out how we'll manage our dependencies, and choose our build tools. None of this is set in stone—the idea is to get going quickly, but at the same time, establish some norms so that transforming our skeleton architecture into application code is as seamless as possible.
Directory structure
The directory structure used to start building our skeleton doesn't need to be fancy. It's a skeleton architecture, not the complete architecture, so the initial directory structure should follow suit. Having said that, we also don't want to use a directory structure that's difficult to evolve into what's actually used in the product. Let's take a look at the items that we'll find in the root of our project directory:

Pretty simple right? Let's walk through what each of these items represent:
main.js
: This is the main entry point into the application. This JavaScript module will bootstrap the initial actions of the system.dispatcher.js
: This is our dispatcher module. This is where the Flux dispatcher instance is created.actions
: This directory contains all our action creator functions and action constants.stores
: This directory contains our store modules.views
: This directory contains our view modules.
This may not seem like much, and this is by design. The directory layout is reflective of the architectural layers of Flux. Obviously there will be more to the actual application once we move past the skeleton architecture phase, but not a whole lot. We should refrain from adding any extraneous components at this point though, because the skeleton architecture is all about information design.
Dependency management
As a starting point, we're going to require the basic Facebook Flux dispatcher as a dependency of our skeleton architecture—even if we don't end up using this dispatcher in our final product. We need to start designing our stores, as this is the most crucial and the most time-consuming aspect of the skeleton architecture; worrying about things like the dispatcher at this juncture simply doesn't pay off.
We need to start somewhere and the Facebook dispatcher implementation is good enough. The question is, will we need any other packages? In Chapter 1, What is Flux? we walked through the setup of the Facebook Flux NPM package and used Webpack to build our code. Can this work as our eventual production build system?
Not having a package manager or a module bundler puts us at a disadvantage, right from the onset of the project. This is why we need to think about dependency management as a first step of the skeleton architecture, even though we don't have many dependencies at the moment. If this is the first time we're building an application that has a Flux architecture behind it, the way we handle dependencies will serve as a future blueprint for subsequent Flux projects.
Is it a bad idea to add more module dependencies during the development of our skeleton architecture? Not at all. In fact, it's better that we use a tool that's well suited for the job. As we're implementing the skeleton, we'll start to see places in our stores where a library would be helpful. For example, if we're doing a lot of sorting and filtering on data collections and we're building higher-order functions, using something like lodash for this is perfect.
On the other hand, pulling in something like ReactJS or jQuery at this stage doesn't make a whole lot of sense because we're still thinking about the information and not how to present it in the DOM. So that's the approach we're going to use in this book—NPM as our package manager and Webpack as our bundler. This is the basic infrastructure we need, without much overhead to distract us.