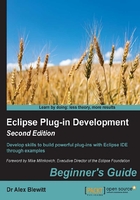
Time for action – getting colorful
To add an option for the ClockWidget
to have a different color, an instance must be obtained instead of the hardcoded BLUE
reference. Since Color
objects are Resource
objects, they must be disposed correctly when the widget is disposed.
To avoid passing in a Color
directly, the constructor will be changed to take an RGB
value (which is three int
values), and use that to instantiate a Color
object to store for later. The lifetime of the Color
instance can be tied to the lifetime of the ClockWidget
.
- Add a private final
Color
field calledcolor
to theClockWidget
:private final Color color;
- Modify the constructor of the
ClockWidget
to take anRGB
instance, and use it to instantiate aColor
object. Note that the color is leaked at this point, and will be fixed later:public ClockWidget(Composite parent, int style, RGB rgb) { super(parent, style); // FIXME color is leaked! this.color = new Color(parent.getDisplay(), rgb); ...
- Modify the
drawClock
method to use this custom color:protected void drawClock(PaintEvent e) { ... e.gc.setBackground(color); e.gc.fillArc(e.x, e.y, e.width-1, e.height-1, arc-1, 2);
- Finally, change the
ClockView
to instantiate the three clocks with different colors:public void createPartControl(Composite parent) { ... final ClockWidget clock = new ClockWidget(parent, SWT.NONE, new RGB(255,0,0)); final ClockWidget clock2 = new ClockWidget(parent, SWT.NONE, new RGB(0,255,0)); final ClockWidget clock3 = new ClockWidget(parent, SWT.NONE, new RGB(0,0,255));
- Now run the application and see the new colors in use:
What just happened?
The Color
was created based on the red, green, blue value passed into the ClockWidget
constructor. Since the RGB
is just a value object (it isn't a Resource
), it doesn't need to be disposed afterwards.
Once the Color
is created, it is assigned to the instance field. When the clocks are drawn, the second hands are in the appropriate colors.
Note
One problem with this approach is that the Color
instance is leaked. When the view is disposed, the associated Color
instance is garbage-collected, but the resources associated with the native handle are not.