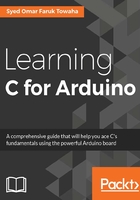
Data types
In our day-to-day life, we use numbers, alphabets, words, and sentences. These can be defined as data. Again, numbers can be pided into two types: fraction and non-fraction, or integer and non-integer. Alphabets and symbols can be defined as characters. Let's look at what we call them in C, and their memory allocation. I will explain what memory allocation is and how you can find out how much memory a data type is consuming later.
Integers
Numbers that can be written without a fractional part are called integers: 5, 28, 273, 100, 986,343 are integers. There are negative integers too: -56, -87, -23,453, -1,000, and so on. They are called int in C programming. If we want to set a variable (number) equal to an integer (here, 24) we can write the following:
int number = 24;
An integer occupies 2 bytes (16 bits) or 4 bytes (32 bits) of memory depending on the processor's architecture. On 32-bit computers, an integer occupies 2 bytes of memory, and on 64-bit computers, integer occupies 4 bytes of memory. For an Arduino Uno, an integer is 2 bytes. A 2-byte integer can hold a number between 2-15 to 215-1 (-32,768 to 32,767). A 4-byte integer can hold a number between 2-31 to 231-1 (-2,147,483, 648 to 2,147,483,647). If a longer number is needed there is another data type in C, named long int or long long int, which can hold longer numbers.
Rational numbers or fractions
In C programming, there are two types of rational numbers. We call them float or double. A float usually occupies shorter memory than a double number. In 64-bit computers, a float can occupy 4 bytes (32 bits) and a double number can occupy 8 bytes (64 bits). Floating numbers are real numbers, as are double numbers. The range of floating numbers is in between ±1.18×10−38 to ±3.4×1038,and the double number ranges between ±2.23×10−308 to ±1.80×10308. So, you can easily imagine how large a number they can hold in their memory. On an Arduino Uno, both float and double occupy 4 bytes (32 bits) of memory. We will use a float type throughout the entire book when necessary.
Say we have two floating numbers and three double numbers, and the variable names for floats are myCGPA and bookPrice; for double numbers, the variables are distance, radius, and length.
So, we can define our floating and double numbers as follows:
float myCGPA = 3.89; float bookPrice = 129.99; double distance = 53523457.435346; double radius = 11e+23; double length = 987902.34532532;
Characters and strings
You might remember when you started learning to read. First you learned the alphabet. Then you learned how to construct a word using the alphabet. And finally, you learned how to make long sentences. The same process applies when you need to learn a programming language. You need to know the alphabets (in C, they are called characters; in short, char), words, or sentences (we can call this a string, or collection of characters). The character data type can hold only a single symbol or alphabet. Say you need to store a letter (here, c) on a variable name, language. You need to define the variable as follows:
char language = 'c';
You cannot assign multiple letters to a character variable. Let's look at an example. You cannot write the following things:
char myword = 'Abracadabra'; char testVar = 'Hi, this is me!';
If you write the preceding statement to declare your character variable on an IDE, the program will not usually show you an error, but if you look at the output of the variables, you will see only the last character. Let's make it clear. If you want to print the preceding two variables in C, your output will show you only 'a' and '!' for the myword and testVar variables, respectively. I will explain why you will get these outputs later. So, we can only store a single character on a char type variable.
Strings are a collection of characters. A sentence or word can be stored in a string variable. C does not have the data type String; people use an array of characters to declare a string in C, but on Arduino Uno, there is string. Let's define a String:
String myText = "This is a lovely day!";
On Arduino IDE, you can write the following:
Char myText[] = "This is a lovely day!";
A character data type in C occupies 1 byte (8 bits) of memory. So, you can easily calculate that, if your word consists of six letters/characters, the string variable would consume 1×6 = 6 bytes of memory; but we must also add an invisible byte that indicates the end of the string with a special null character, to reach 7 bytes.
Note
Have you noticed that, when we declared our character type variables, we used single quotes, and when we declared our sting type variables we used double quotes? Yes, this is the rule for defining a character and a string. A character need to be inside single quotes and a string needs to be in between double quotes.
Every character has an ASCII number. You can call the character by its ASCII number.
Character A has an ASCII number of 65. We can also print character A by writing the following code:
void setup() { Serial.begin(9600); Serial.write(65); } void loop() { }

Have you noticed we used Serial.write()
instead of Serial.print()
? Yes, this is how we print a byte in C for Arduino.
You can test printing other bytes too. Remember, the limit of character bytes is from 33-126 (visible characters). Go to File
| Example
| 04. Communication
| ASCIITable
. Upload the code to the Arduino Board; you will get full details of all the valid characters in a table.
Note
ASCII is the short form of American Standard Code for Information Exchange. ASCII is used in raw coding and fast coding. We sometimes need to use characters or strings in our programming, but our computer only understands numbers. So, it converts the characters or string into numbers. The ASCII code is the numerical representation of a character. By 65, in ASCII, we mean the character A (capital letter); by 97, we mean character a (lowercase letter).
Booleans
A Boolean is another type of data type in C for Arduino. It represents if something is true or false. In Arduino IDE, we declare a Boolean data type as follows:
boolean testVar;
Or:
bool testVar;
The values of the data type can be assigned as 0 or 1 and true or false (watch the case: False/True instead of false/true will not work). The output of the code will always be 0
or 1
. The following shows a full list of how to declare a Boolean variable:
bool testbool1 = 0; bool testbool2 = 1; bool testbool3 = true; bool testbool4 = false; boolean mybool1 = 0; boolean mybool2 = 1; boolean mybool3 = true; boolean mybool4 = false;
Let's look at an example. This is not a perfect example. We will look at more usages of Boolean data types in the next chapter.
The following code will print the binary numbers (0
or 1
):
bool testbool1 = 0; bool testbool2 = 1; bool testbool3 = true; bool testbool4 = false; boolean mybool1 = 0; boolean mybool2 = 1; boolean mybool3 = true; boolean mybool4 = false; void setup() { Serial.begin(9600); Serial.println(testbool1); Serial.println(testbool2); Serial.println(testbool3); Serial.println(testbool4); Serial.println(mybool1); Serial.println(mybool2); Serial.println(mybool3); Serial.println(mybool4); } void loop() { }
The output of the code will look like the following screenshot:

See, only binary numbers are printed (no true or false).
There are other data types in C for Arduino, too. Some of them are byte, array, word, void, and so on. We will use them if we really have to. When we use them, we will also give some descriptions for them. First, we need to know the basic variables and their usages.
Let's learn about all the data types using Arduino IDE.