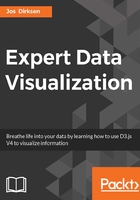
Binding the data and updating existing rectangles
The next step is assigning this data to a D3 selection. We do this by using the selectAll function on the chart variable we defined earlier (remember this is the main g element, we added initially):
var rectangles = chart.selectAll("rect").data(data);
This call will select all the rectangles which are already appended as children to the chart variable. The first time this is called, rectangles will have no children, but on subsequent calls this will select any rectangles that have been added in the previous call to the update() function. To differentiate between newly added rectangles and rectangles which we'll reuse, we add a specific CSS class. Besides just adding the CSS class, we also need to make sure they have the correct width and height properties set, since the bound data has changed.
In the case of rectangles which we reuse, we do that like this:
rectangles.attr("class", "update")
.attr("width", function(d) {return d})
.attr("height", function(d) {return d});
To set the CSS we use the attr function to set the class property, which points to a style defined in our CSS file. The width and height properties are set in the same manner, but their value is based on the value of the passed data. You can do this by setting the value of that attribute to a function(d) {...}. The d which is passed in to this function is the value of the corresponding element from the bound data array. So the first rectangle which is found is bound to data[0], the second to data[1], and so on. In this case, we set both the width and the height of the rectangle to the same value.
The CSS for this class is very simple, and just makes sure that the newly added rectangles are filled with a nice blue color:
.update {
fill: steelblue;
}